Intersection Types In TypeScript
Updated on · 4 min read|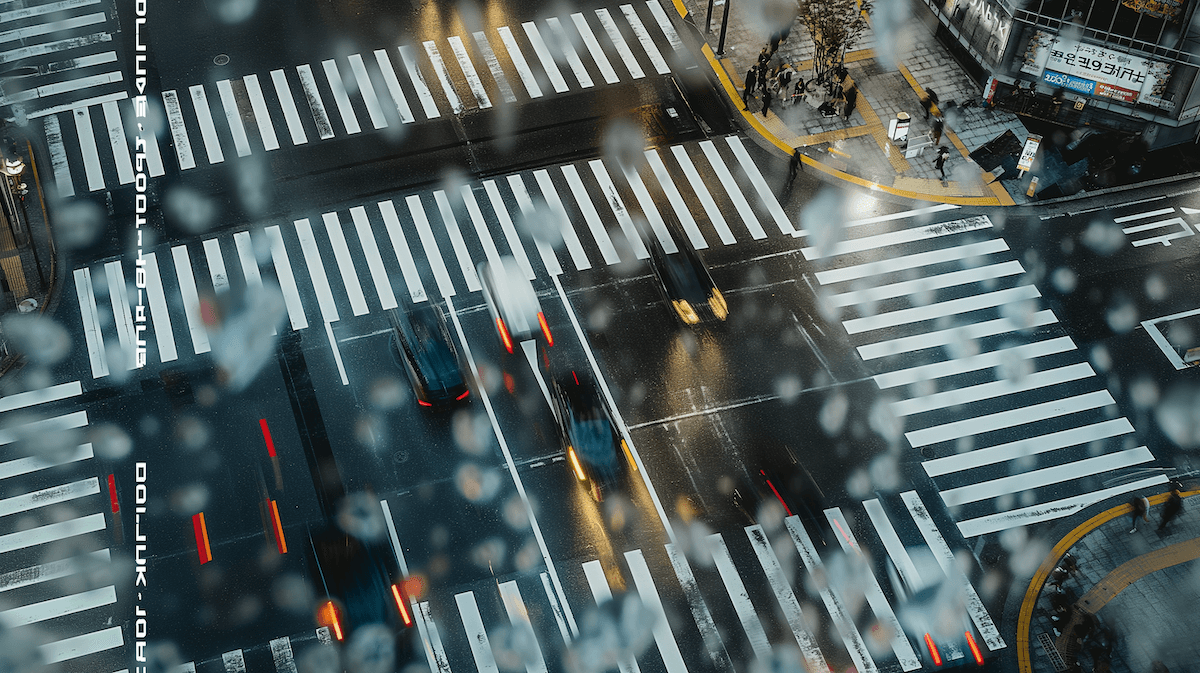
When it comes to structuring complex type relationships in TypeScript, intersection types are one of the basic tools in a developer's arsenal. They provide a way of combining multiple types into one, enabling the creation of sophisticated type systems that can enhance the development workflow and maintainability of the code.
In contrast to union types, which allow for flexibility in the shape of a variable, intersection types require that a variable meets all the criteria of the combined types. This can be useful when you need to ensure that a variable has all the properties of multiple types or when you want to create a new type that combines the features of existing ones.
In this post, we'll explore the basics of intersection types in TypeScript and how they can be used to create more robust type systems. We'll cover the syntax for defining intersection types, how they interact with other types, and some common use cases where they can be beneficial.
Intersection types in theory
Definition and syntax
Intersection types allow developers to combine multiple types into one. This is done using the &
symbol, which is placed between the types that are to be combined. The resulting type has all the properties of the combined types. Intersection types are particularly useful when there is a need to merge several typings into one entity.
The syntax for an intersection type is straightforward. For example, if TypeA
and TypeB
are two different types, their intersection is represented as TypeA & TypeB
. The resulting type will have all members of TypeA
and TypeB
.
Basic examples
To demonstrate the simplicity and effectiveness of intersection types, consider the following basic example:
typescripttype Name = { firstName: string; lastName: string; }; type Birthdate = { birthDate: Date; }; // Intersection type of Name and Birthdate type Person = Name & Birthdate; // Person has properties from both Name and Birthdate const person: Person = { firstName: "Jane", lastName: "Doe", birthDate: new Date("1990-01-01"), };
typescripttype Name = { firstName: string; lastName: string; }; type Birthdate = { birthDate: Date; }; // Intersection type of Name and Birthdate type Person = Name & Birthdate; // Person has properties from both Name and Birthdate const person: Person = { firstName: "Jane", lastName: "Doe", birthDate: new Date("1990-01-01"), };
In this example, Person
is an intersection type that combines Name
and Birthdate
. Any object of type Person
will be required to have all properties of both Name
and Birthdate
, as seen in the person
object. This is a powerful feature for building composite types that suit specific needs without having to duplicate code or create unnecessary class hierarchies.
Intersection types can also be used to mix in functionality or state from several sources, resulting in a more cohesive codebase where separation of concerns can be maintained, yet combined types can be composed as needed.
Practical applications of intersection types in TypeScript
Designing function signatures for library authors
One practical application of intersection types is in the design of comprehensive function signatures by library authors. By using intersection types, authors can create functions that accept a wide range of parameters while maintaining strict typing and enhancing the API's usability.
To illustrate this with an example, consider a logging function designed to handle both log messages and errors:
typescripttype LogMessage = { message: string; }; type LogError = { error: Error; }; // Function that accepts an intersection of LogMessage and LogError function logEvent(payload: LogMessage & LogError): void { console.log(payload.message); console.error(payload.error); } // Usage logEvent({ message: "Log in attempt", error: new Error("Login failed") }); // Type error: Property error is missing in type { message: string; } // but required in type LogError logEvent({ message: "User logged in" });
typescripttype LogMessage = { message: string; }; type LogError = { error: Error; }; // Function that accepts an intersection of LogMessage and LogError function logEvent(payload: LogMessage & LogError): void { console.log(payload.message); console.error(payload.error); } // Usage logEvent({ message: "Log in attempt", error: new Error("Login failed") }); // Type error: Property error is missing in type { message: string; } // but required in type LogError logEvent({ message: "User logged in" });
In the above example, logEvent
expects a payload that has both a message
and an error
. It's a strict contract; callers must provide an object that meets both LogMessage
and LogError
properties.
By contrast, if the function signature used a union type, the signature would look like this:
typescript// Using union types function logEvent(payload: LogMessage | LogError): void { if ("message" in payload) { console.log(payload.message); } if ("error" in payload) { console.error(payload.error); } }
typescript// Using union types function logEvent(payload: LogMessage | LogError): void { if ("message" in payload) { console.log(payload.message); } if ("error" in payload) { console.error(payload.error); } }
In this case, we need to explicitly check for the presence of each property as both of them are optional.
Enhancing type safety in application development
Intersection types play a crucial role in enhancing type safety. They ensure objects conform to multiple type constraints, which is particularly useful in complex applications with layered architectures.
Consider a scenario where a component requires props that are a combination of different aspects:
typescripttype WithLoadingState = { isLoading: boolean; }; type WithErrorState = { error?: Error; }; // Ensuring the component props include both loading and error state type ComponentProps = WithLoadingState & WithErrorState; function MyComponent(props: ComponentProps) { // The component has access to both the loading state and the error state }
typescripttype WithLoadingState = { isLoading: boolean; }; type WithErrorState = { error?: Error; }; // Ensuring the component props include both loading and error state type ComponentProps = WithLoadingState & WithErrorState; function MyComponent(props: ComponentProps) { // The component has access to both the loading state and the error state }
Intersection types and type guards
Intersection types can be utilized alongside type guards to implement runtime type-checks that narrow down the type of a variable within a code block. This can be particularly useful when dealing with code branches where the exact type may differ:
typescripttype Worker = { id: number; schedule: string[]; }; type Student = { id: number; courses: string[]; }; // An object representing a part-time student who also works type WorkingStudent = Worker & Student; // A custom type guard to check if an object is a WorkingStudent function isWorkingStudent(person: object): person is WorkingStudent { return "schedule" in person && "courses" in person; } function printSchedule(person: object): void { if (isWorkingStudent(person)) { // If the person is a WorkingStudent, we can access both schedule and courses console.log("Work Schedule: ", person.schedule); console.log("Course List: ", person.courses); } }
typescripttype Worker = { id: number; schedule: string[]; }; type Student = { id: number; courses: string[]; }; // An object representing a part-time student who also works type WorkingStudent = Worker & Student; // A custom type guard to check if an object is a WorkingStudent function isWorkingStudent(person: object): person is WorkingStudent { return "schedule" in person && "courses" in person; } function printSchedule(person: object): void { if (isWorkingStudent(person)) { // If the person is a WorkingStudent, we can access both schedule and courses console.log("Work Schedule: ", person.schedule); console.log("Course List: ", person.courses); } }
Using intersection types in combination with type guards empowers developers to write robust, type-safe functions that can handle different structures and scenarios effectively. This amalgamation of features is a testament to TypeScript's ability to model complex type relationships and ensure type correctness throughout a codebase.
Conclusion
Intersection types are a powerful feature of TypeScript that enables developers to create more robust and expressive type systems. By combining multiple types into one, developers can define complex relationships between types and ensure that variables meet specific criteria. This can lead to more maintainable code, better type safety, and improved developer productivity.
In this post, we explored the basics of intersection types in TypeScript, including their syntax, use cases, and practical applications. By leveraging intersection types effectively, developers can build more sophisticated type systems that enhance the quality and reliability of their code. Whether designing function signatures, enhancing type safety, or implementing type guards, intersection types are a valuable tool in a TypeScript developer's toolkit.