Higher-Order Functions In JavaScript
Updated on · 6 min read|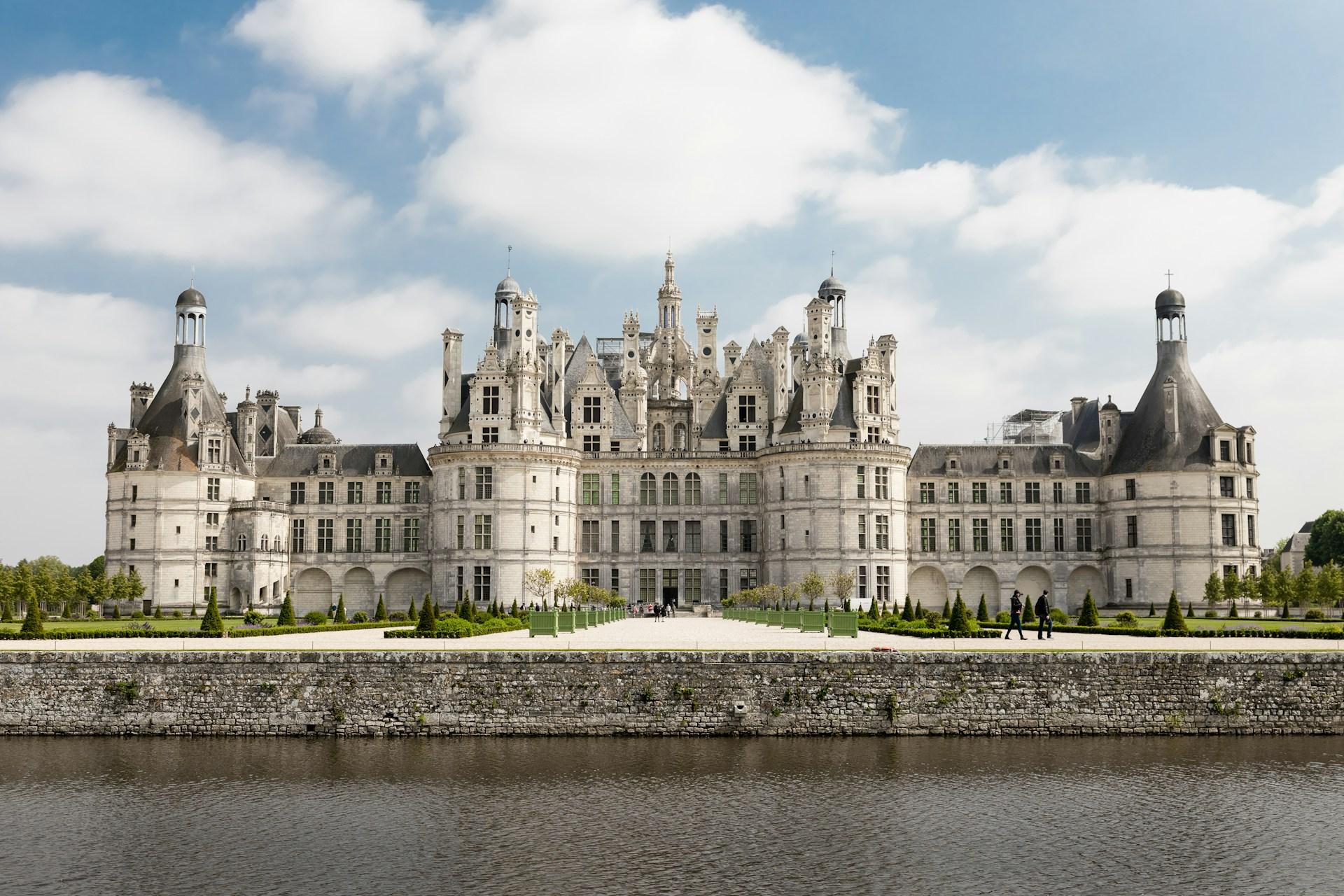
JavaScript's functional programming features offer developers powerful tools to write more expressive and maintainable code. Among these tools, higher-order functions are particularly valuable, serving as a foundation for many coding patterns and techniques used in modern development practices. They enable the abstraction of control flows and data operations, which are essential for creating scalable and robust applications.
Higher-order functions are an elegant aspect of JavaScript that allows for transforming, filtering, or combining data with ease. This post will explore the concept of higher-order functions, providing clear examples of their use in everyday coding. From the commonly used built-in methods like map
, filter
, and reduce
to the creation of custom higher-order functions that enhance functionality, this article will shed light on the power and flexibility of this important feature in JavaScript.
Understanding higher-order functions
Higher-order functions stand as a cornerstone in the JavaScript language, serving as a defining feature of its functional programming paradigm. These functions enhance the language's capabilities, enabling developers to write more concise and expressive code.
Defining higher-order functions
In JavaScript, a higher-order function is one that either accepts another function as an argument, returns a function as its result, or both. This concept is not exclusive to JavaScript and is prevalent in many other programming languages, especially those that support functional programming.
javascript// Example of a higher-order function that takes a function as an argument function repeatTwice(action) { action(); action(); } // Usage function sayHello() { console.log("Hello!"); } repeatTwice(sayHello); // Output: Hello! Hello!
javascript// Example of a higher-order function that takes a function as an argument function repeatTwice(action) { action(); action(); } // Usage function sayHello() { console.log("Hello!"); } repeatTwice(sayHello); // Output: Hello! Hello!
These functions are more than just a syntactical convenience; they allow developers to abstract logic and create more generic, reusable code. For instance, a function that operates on arrays can be designed to accept a function that describes the operation to be performed on each element, rather than hardcoding the operation within the function itself.
Importance in JavaScript programming
The critical role of higher-order functions in JavaScript cannot be understated. They enable developers to build abstractions that can manipulate behavior at runtime, which opens up a landscape of possibilities from improving code modularity to enhancing the expressiveness of the language.
One of the main benefits of higher-order functions is that they can help avoid code repetition by generalizing actions. This generalization leads to less verbose and more manageable code, making it easier to understand and debug. It allows for the development of libraries and frameworks that offer high levels of customization while keeping the code base DRY (Don't Repeat Yourself).
javascript// Example of a higher-order function that returns a function function greaterThan(n) { return function (m) { return m > n; }; } let greaterThan10 = greaterThan(10); console.log(greaterThan10(12)); // Output: true console.log(greaterThan10(8)); // Output: false
javascript// Example of a higher-order function that returns a function function greaterThan(n) { return function (m) { return m > n; }; } let greaterThan10 = greaterThan(10); console.log(greaterThan10(12)); // Output: true console.log(greaterThan10(8)); // Output: false
Additionally, the use of higher-order functions often leads to a declarative programming style, which can be easier to reason about than an imperative style. Instead of describing how to perform tasks with loops and conditional statements, a declarative style focuses on what should be done by utilizing functions that encapsulate these actions.
Higher-order functions also create a foundation for patterns and techniques such as currying and function composition, which are powerful tools for functional programming in JavaScript. Through their ability to manipulate functions as first-class citizens, they foster a functional approach to problem-solving that can significantly reduce side effects and improve code predictability.
javascript// Composition of higher-order functions function compose(f, g) { return function (value) { return f(g(value)); }; } function addOne(num) { return num + 1; } function square(num) { return num * num; } let addOneAndSquare = compose(square, addOne); console.log(addOneAndSquare(4)); // Output: 25 ((4+1)^2)
javascript// Composition of higher-order functions function compose(f, g) { return function (value) { return f(g(value)); }; } function addOne(num) { return num + 1; } function square(num) { return num * num; } let addOneAndSquare = compose(square, addOne); console.log(addOneAndSquare(4)); // Output: 25 ((4+1)^2)
Additionally, with the introduction of arrow functions in JavaScript, the syntax for defining functions has become more concise and expressive. Arrow functions provide a shorthand syntax for writing function expressions, making them particularly suitable for use as arguments in higher-order functions like those demonstrated above. For instance, the compose function can be redefined using arrow functions as follows:
javascript// Composition of higher-order functions using arrow functions const compose = (f, g) => (value) => f(g(value)); const addOne = (num) => num + 1; const square = (num) => num * num; const addOneAndSquare = compose(square, addOne); console.log(addOneAndSquare(4)); // Output: 25 ((4+1)^2)
javascript// Composition of higher-order functions using arrow functions const compose = (f, g) => (value) => f(g(value)); const addOne = (num) => num + 1; const square = (num) => num * num; const addOneAndSquare = compose(square, addOne); console.log(addOneAndSquare(4)); // Output: 25 ((4+1)^2)
Common higher-order functions in JavaScript
JavaScript comes with a suite of built-in higher-order functions, especially for array manipulation. These functions provide an elegant way to handle common tasks involving collections, and they are frequently used because of their utility and convenience.
Array.prototype.map
The map
method is one of the quintessential higher-order functions in JavaScript. It takes a callback function as an argument and returns a new array that is the result of applying this callback to every element in the original array. The beauty of map
lies in its immutability and its ability to apply a transformation to an array without altering the original data.
javascript// Using map to double the values in an array const numbers = [1, 2, 3, 4]; const doubledNumbers = numbers.map((number) => number * 2); console.log(doubledNumbers); // Output: [2, 4, 6, 8]
javascript// Using map to double the values in an array const numbers = [1, 2, 3, 4]; const doubledNumbers = numbers.map((number) => number * 2); console.log(doubledNumbers); // Output: [2, 4, 6, 8]
Array.prototype.filter
filter
is another commonly used higher-order function that creates a new array containing all the elements that pass a test implemented by the provided function. This is particularly useful for conditional filtering of array elements.
javascript// Filtering out numbers less than 3 const numbers = [1, 2, 3, 4, 5]; const filteredNumbers = numbers.filter((number) => number >= 3); console.log(filteredNumbers); // Output: [3, 4, 5]
javascript// Filtering out numbers less than 3 const numbers = [1, 2, 3, 4, 5]; const filteredNumbers = numbers.filter((number) => number >= 3); console.log(filteredNumbers); // Output: [3, 4, 5]
Array.prototype.reduce
Perhaps the most powerful array method, reduce
, takes a callback function and an optional initial value to progressively accumulate the array elements into a single value. This method is highly versatile and can be used for a wide range of purposes, from summing numbers to flattening nested arrays. In fact, map
and filter
can be implemented using reduce
, which demonstrates its expressive power.
javascript// Summing up the elements of an array using reduce const numbers = [1, 2, 3, 4]; const sum = numbers.reduce( (accumulator, currentValue) => accumulator + currentValue, 0, ); console.log(sum); // Output: 10
javascript// Summing up the elements of an array using reduce const numbers = [1, 2, 3, 4]; const sum = numbers.reduce( (accumulator, currentValue) => accumulator + currentValue, 0, ); console.log(sum); // Output: 10
These are just a few examples of the commonly used higher-order functions in JavaScript. The philosophy behind these functions is centered on providing a declarative approach to array manipulations, abstracting away the need to manually iterate over data structures and operate on their elements. Whether through transformation, filtering, or accumulation, these functions present a more expressive and powerful way to handle common tasks with improved readability and maintainability.
Creating custom higher-order functions
JavaScript not only provides built-in higher-order functions, but it also allows developers to craft their own, catering to the unique needs of their applications. Custom higher-order functions can enhance the language's expressiveness and provide tailored solutions to complex problems.
Writing functions that accept callbacks
Developers can define their own higher-order functions to take callbacks, offering a versatile way to incorporate user-defined behavior. A primary benefit of such functions is their adaptability to various contexts and requirements.
javascriptfunction processArrayItems(array, actionCallback, filterCallback) { return array .filter(filterCallback ? filterCallback : () => true) .map(actionCallback); } // Usage const numbers = [1, 2, 3, 4, 5]; const isEven = (number) => number % 2 === 0; const square = (number) => number * number; const processedNumbers = processArrayItems(numbers, square, isEven); console.log(processedNumbers); // Output: [4, 16]
javascriptfunction processArrayItems(array, actionCallback, filterCallback) { return array .filter(filterCallback ? filterCallback : () => true) .map(actionCallback); } // Usage const numbers = [1, 2, 3, 4, 5]; const isEven = (number) => number % 2 === 0; const square = (number) => number * number; const processedNumbers = processArrayItems(numbers, square, isEven); console.log(processedNumbers); // Output: [4, 16]
In this example, processArrayItems
is a higher-order function that accepts an array
to process, an actionCallback
to apply to each element, and an optional filterCallback
to determine which elements should be processed. The square
function is used to square the number, and isEven
serves as the filter to only apply square
to even numbers. This provides a flexible setup where the caller can plug in different actions and filters without changing the underlying iteration logic.
Returning functions from functions
Higher-order functions can also return other functions. This technique can be used to create partially applied functions or to encapsulate functionality within a specific scope. Returning functions from functions is a powerful concept for achieving modular and composable code.
javascript// A custom higher-order function that captures and retains a condition function notMatchingCondition(condition) { return function (item) { return !condition(item); }; } // Usage const numbers = [1, 2, 3, 4, 5]; const isOdd = (number) => number % 2 !== 0; const rejectOddNumbers = numbers.filter(notMatchingCondition(isOdd)); console.log(rejectOddNumbers); // Output: [2, 4]
javascript// A custom higher-order function that captures and retains a condition function notMatchingCondition(condition) { return function (item) { return !condition(item); }; } // Usage const numbers = [1, 2, 3, 4, 5]; const isOdd = (number) => number % 2 !== 0; const rejectOddNumbers = numbers.filter(notMatchingCondition(isOdd)); console.log(rejectOddNumbers); // Output: [2, 4]
In this example, notMatchingCondition
is a higher-order function that returns a function. This returned function captures the condition
and negates it, effectively creating a new condition that can be used to filter out elements from an array. This pattern is particularly useful for creating reusable predicates that can be composed and combined to form more complex logic.
Conclusion
In the landscape of JavaScript, higher-order functions play an essential role in crafting modern and efficient code. These functions enhance the language's functionality, making it a powerful tool for developers to express complex logic in a concise and readable manner.
The use of both built-in and custom higher-order functions leads to a declarative programming style, where the intent of the code is clear and the mechanisms are abstracted away. This approach minimizes side effects and encourages immutable data patterns, which are key concepts in functional programming that contribute to the stability and reliability of software.