Error Handling and Defensive Programming with TypeScript
Updated on · 7 min read|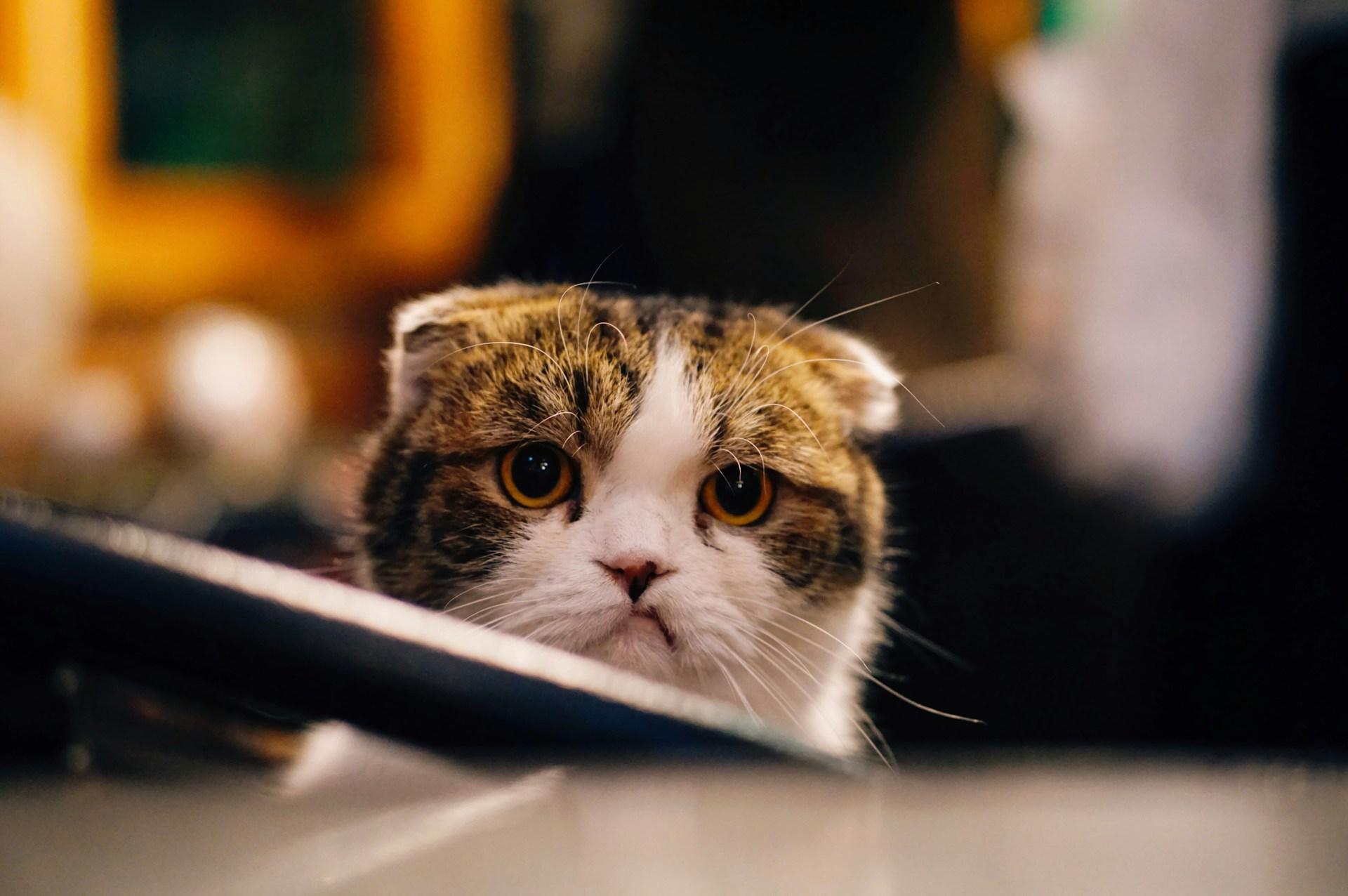
Errors are an inseparable part of software development, arising from unforeseen circumstances such as invalid user input, server failures, or bugs in the code. Error handling refers to the process of anticipating, detecting, and resolving these problems to maintain a smooth user experience and prevent application crashes. Defensive programming is a complementary approach, intended to mitigate errors by writing code that's robust against potential misuse and unforeseen scenarios.
TypeScript, a superset of JavaScript, has gained significant popularity due to its static typing and advanced features, which enable developers to catch errors at compile-time rather than at runtime. This not only makes the code more reliable but also enhances developer productivity by flagging potential issues early in the development cycle.
In this post, we will explore how TypeScript's type system and compiler options can be leveraged for effective error handling and defensive programming. We'll examine common error types in TypeScript, its exception handling mechanisms, and provide practical techniques to write more resilient code. By the end of this post, developers will be equipped with strategies to anticipate and prevent errors, ensuring their applications are secure, reliable, and maintainable.
Understanding errors in TypeScript
Grasping the nature of errors in TypeScript is fundamental to implementing effective error handling. Errors in TypeScript can be broadly categorized into two types: compile-time errors and runtime errors.
-
Compile-time errors occur while the code is being transformed from TypeScript to JavaScript by the TypeScript compiler. These include syntax errors, type errors, and other issues that can be detected before the code is executed.
-
Runtime errors, on the other hand, are errors that escape this compilation process and only occur once the JavaScript code is running. These can be caused by faulty logic, unexpected user input, or external system failures that the compiler couldn't predict.
TypeScript's strict type system is designed to catch a wide array of error types at compile-time. Some of the common errors that TypeScript developers encounter include:
- Type errors - when a value does not match the expected type.
- Null and undefined errors - when objects that are expected to be defined or contain a non-null value are otherwise.
- Scope errors - when variables or functions are used outside their accessible scope.
- Syntax errors - when the code written does not comply with the rules of the language.
Defensive programming techniques in TypeScript
Defensive programming in TypeScript is about writing code that proactively prevents errors rather than simply reacting to them. It's about anticipating possible problems and coding to avoid these issues before they happen. Here are some defensive programming techniques specific to TypeScript that help in building robust and less error-prone applications:
Input validation and type guards
One of the most critical defensive programming practices is to validate inputs before using them in your program. TypeScript's type system significantly aids in this by ensuring that variables and parameters are of the expected type. However, when dealing with any external input that TypeScript can't verify at compile time - like user input, API responses, or file contents - it's essential to validate data at runtime:
typescriptfunction processInput(input: any) { if (typeof input === "string") { // valid input, continue processing } else { // invalid input, throw error or handle accordingly } }
typescriptfunction processInput(input: any) { if (typeof input === "string") { // valid input, continue processing } else { // invalid input, throw error or handle accordingly } }
Type guards are a powerful feature in TypeScript for input validation. They allow you to ensure a value matches a particular type:
typescriptfunction isNumber(value: unknown): value is number { return typeof value === "number"; }
typescriptfunction isNumber(value: unknown): value is number { return typeof value === "number"; }
Function and method preconditions
Preconditions are checks performed at the beginning of a function or method to ensure that the inputs and the state of the system are valid before proceeding with further logic. For instance:
typescriptfunction divide(dividend: number, divisor: number): number { if (divisor === 0) { throw new Error("Cannot divide by zero."); } return dividend / divisor; }
typescriptfunction divide(dividend: number, divisor: number): number { if (divisor === 0) { throw new Error("Cannot divide by zero."); } return dividend / divisor; }
These checks help avoid errors due to incorrect arguments or improper system state, ensuring that the function can operate safely on the inputs provided. TypeScript's type system can be used to enforce these preconditions, making it clear what inputs are expected and what the function guarantees in return.
Immutable data structures with readonly
Immutability is a principle that helps in writing predictable code. If objects cannot be modified after their creation, it prevents a whole class of errors related to unexpected changes. TypeScript supports immutability through the readonly
keyword:
typescriptclass Point { readonly x: number; readonly y: number; constructor(x: number, y: number) { this.x = x; this.y = y; } } const p = new Point(1, 2); p.x = 3; // Error: cannot assign to 'x' because it is a read-only property.
typescriptclass Point { readonly x: number; readonly y: number; constructor(x: number, y: number) { this.x = x; this.y = y; } } const p = new Point(1, 2); p.x = 3; // Error: cannot assign to 'x' because it is a read-only property.
By using readonly
, you can ensure certain properties of your classes and interfaces are not changed inadvertently, leading to fewer side effects and better maintenance over time.
Defensive copying and partial types
When working with complex data structures, it's often beneficial to create defensive copies of objects to prevent unexpected changes. TypeScript's Partial
type can be used to create a new type that represents the same structure as another type, but with all properties set to optional:
typescriptinterface User { id: string; name: string; age: number; } function updateUser(user: User, updates: Partial<User>): User { return { ...user, ...updates }; }
typescriptinterface User { id: string; name: string; age: number; } function updateUser(user: User, updates: Partial<User>): User { return { ...user, ...updates }; }
By using Partial
, you can ensure that the original object is not modified and that the updates are applied in a safe and controlled manner. This is especially useful when working with state management and data transformations.
Defensive programming is inherently about being cautious and thoughtful when writing code. By using TypeScript's type safety, implementing runtime checks where necessary, and adhering to immutable structures wherever possible, developers can construct a strong defensive code that resists common errors and aligns with robust software engineering practices.
Handling nullable types and null safety
Handling null
and undefined
values is a common source of errors in JavaScript and TypeScript. Null reference errors, also known as "null pointer exceptions" in other languages, occur when a program tries to access a property or method of a null
or undefined
value. TypeScript provides several features and best practices to manage nullable types and ensure null safety.
Understanding optional chaining and nullish coalescing
Optional chaining (?.
) and nullish coalescing (??
) operators in TypeScript offer concise ways to handle potentially null
or undefined
values without verbose and repetitive checks.
typescriptinterface User { id: string; profile?: { name: string; age?: number; }; } // Optional chaining function getUserName(user: User): string | undefined { return user.profile?.name; } const user: User = { id: "1", profile: { name: "Alice" } }; console.log(getUserName(user)); // "Alice" console.log(getUserName({ id: "2" })); // undefined // Nullish coalescing function getUserAge(user: User): number { return user.profile?.age ?? -1; } console.log(getUserAge(user)); // -1 console.log(getUserAge({ id: "3", profile: { name: "Bob", age: 25 } })); // 25
typescriptinterface User { id: string; profile?: { name: string; age?: number; }; } // Optional chaining function getUserName(user: User): string | undefined { return user.profile?.name; } const user: User = { id: "1", profile: { name: "Alice" } }; console.log(getUserName(user)); // "Alice" console.log(getUserName({ id: "2" })); // undefined // Nullish coalescing function getUserAge(user: User): number { return user.profile?.age ?? -1; } console.log(getUserAge(user)); // -1 console.log(getUserAge({ id: "3", profile: { name: "Bob", age: 25 } })); // 25
Type guards for runtime null checks
Type guards can be used to check not only for data types but also check if a value is not null
, giving developers fine-grained control over null checks at runtime, especially when enhanced by generic types. This is particularly useful when working with external data sources or APIs:
typescriptfunction isNonNull<T>(value: T | null | undefined): value is T { return value !== null && typeof value !== "undefined"; } function getValueOrFallback( value: string | null | undefined, fallback: string, ): string { return isNonNull(value) ? value : fallback; } console.log(getValueOrFallback(null, "default string")); // "default string"
typescriptfunction isNonNull<T>(value: T | null | undefined): value is T { return value !== null && typeof value !== "undefined"; } function getValueOrFallback( value: string | null | undefined, fallback: string, ): string { return isNonNull(value) ? value : fallback; } console.log(getValueOrFallback(null, "default string")); // "default string"
Aliases and type unions for nullable types
Creating aliases for nullable types can improve code readability and maintainability, making it easier to work with types that can be null
or undefined
.
typescripttype MaybeString = string | null | undefined; function logMessage(message: MaybeString) { if (isNonNull(message)) { console.log(message); } else { console.log("No message to display."); } }
typescripttype MaybeString = string | null | undefined; function logMessage(message: MaybeString) { if (isNonNull(message)) { console.log(message); } else { console.log("No message to display."); } }
Through these techniques, TypeScript developers can effectively safeguard against the pitfalls associated with null
and undefined
. By embracing null safety features and best practices, they substantially reduce the frequency of null reference errors and enhance the overall reliability of their applications.
Advanced error handling patterns
TypeScript allows developers to use advanced error handling patterns that can make their code even more robust and maintainable. These patterns take advantage of TypeScript's static typing and advanced type inference capabilities to provide compile-time checks for error handling logic.
Using union types for error handling
TypeScript's union types can be used to represent a value that could be one of several different types, which is useful for functions that might return a result or an error. For example:
typescripttype SuccessResponse = { success: true; value: number }; type ErrorResponse = { success: false; error: string }; function divide( dividend: number, divisor: number, ): SuccessResponse | ErrorResponse { if (divisor === 0) { return { success: false, error: "Cannot divide by zero." }; } return { success: true, value: dividend / divisor }; }
typescripttype SuccessResponse = { success: true; value: number }; type ErrorResponse = { success: false; error: string }; function divide( dividend: number, divisor: number, ): SuccessResponse | ErrorResponse { if (divisor === 0) { return { success: false, error: "Cannot divide by zero." }; } return { success: true, value: dividend / divisor }; }
By checking the success
property, the caller can handle each case appropriately, and TypeScript's type system will ensure the correct fields are accessed for each case.
Implementing discriminated unions for error states
Discriminated unions, also known as tagged unions, are an extension of the union type pattern that makes it even clearer how to handle different cases. Each type in a discriminated union has a common, singleton-type property — typically called kind
or type
. This property makes implementing runtime type guards straightforward, allowing for more readable and safe type discrimination:
typescripttype Result = | { kind: "success"; value: number } | { kind: "failure"; error: Error }; function getResult(): Result { // Some logic that might succeed or fail } const result = getResult(); switch (result.kind) { case "success": console.log(`The result is ${result.value}`); break; case "failure": console.error(`An error occurred: ${result.error.message}`); break; }
typescripttype Result = | { kind: "success"; value: number } | { kind: "failure"; error: Error }; function getResult(): Result { // Some logic that might succeed or fail } const result = getResult(); switch (result.kind) { case "success": console.log(`The result is ${result.value}`); break; case "failure": console.error(`An error occurred: ${result.error.message}`); break; }
Error boundaries and propagation
In complex applications, especially those with nested components or layers, it's helpful to implement error boundaries — structures that catch errors in their subcomponents and prevent them from propagating and affecting higher levels. This pattern is particularly useful in user interface frameworks but can also be used in more general contexts:
typescriptclass ErrorBoundary { try<T>(func: () => T): T | null { try { return func(); } catch (e) { // Handle error and prevent it from propagating this.handleError(e); return null; } } private handleError(error: Error) { // Log the error and possibly recover } } const boundary = new ErrorBoundary(); boundary.try(() => { // Some potentially error-throwing operation });
typescriptclass ErrorBoundary { try<T>(func: () => T): T | null { try { return func(); } catch (e) { // Handle error and prevent it from propagating this.handleError(e); return null; } } private handleError(error: Error) { // Log the error and possibly recover } } const boundary = new ErrorBoundary(); boundary.try(() => { // Some potentially error-throwing operation });
With these advanced error handling patterns, TypeScript developers can write code that elegantly handles different error conditions, making their applications more reliable and their error handling code easier to understand and maintain. These patterns take full advantage of the powerful type system provided by TypeScript, ensuring that errors are not just handled correctly at runtime but are also clearly and explicitly represented in the codebase.
Conclusion
TypeScript's robust type system brings a level of reliability and maintainability to JavaScript projects that was previously difficult to achieve. By understanding and leveraging TypeScript features for error handling and defensive programming, developers can write code that is resilient to many common types of runtime errors.
Defensive programming strategies such as input validation with type guards, enforcing immutability with Readonly
, using Partial
types, managing state with discriminated unions, and implementing type-safe design patterns result in applications that are robust and scalable.
Managing nullable types through optional chaining, nullish coalescing, strict null checks, non-nullable assertions, and runtime null checks helps to avoid one of the most common sources of bugs - the dreaded null reference error.
In summary, TypeScript's static typing features, combined with careful error handling and defensive programming techniques, offer a powerful toolkit for crafting high-quality software. By leveraging these features and patterns, developers can create software that is not only functional but also resilient and easy to maintain, leading to a more enjoyable development experience and higher satisfaction for end-users.