Using Generics In TypeScript: A Practical Guide
Updated on · 7 min read|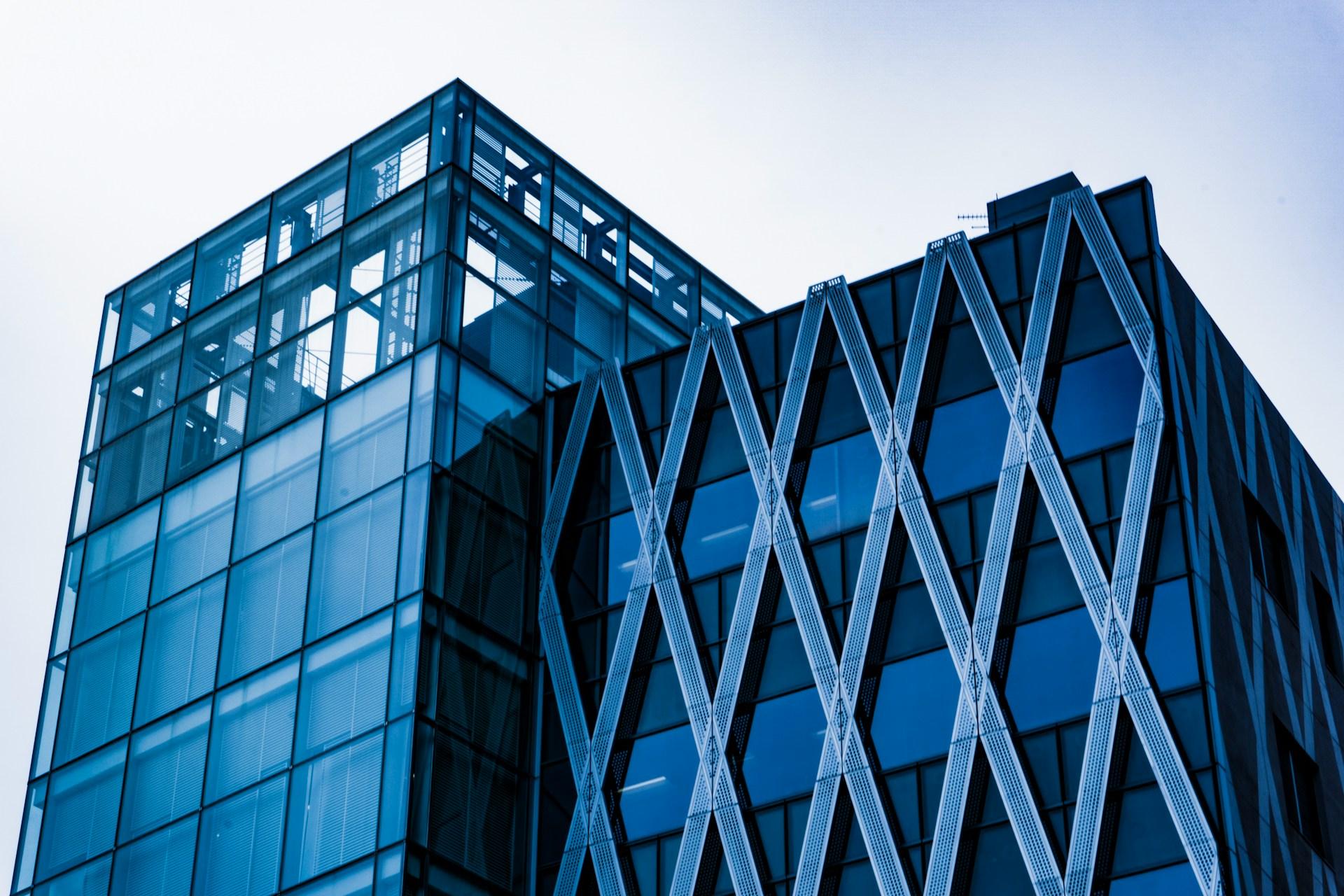
TypeScript, a strongly typed language built on top of JavaScript, has revolutionized the way developers write code for large-scale applications by providing advanced typing features and tools, such as type inference, generics, conditional types, type guards and template literal types. Among these features, generics stand out as one of the most powerful tools for creating flexible, reusable components without sacrificing type safety. In this blog post, we'll dive into the world of generics in TypeScript, exploring how they can be used to write cleaner, more maintainable code that is robust and easy to understand.
Understanding generics in TypeScript
Generics are a fundamental concept not only in TypeScript but in many modern programming languages. They allow developers to write flexible, reusable code by providing a way to pass types as parameters to components such as functions, interfaces, or classes. Essentially, generics enable the creation of a component that can work over a variety of types rather than a single one.
At its core, TypeScript's generic syntax allows defining a type variable in angle brackets (< >
). This type variable can then be used throughout the component (like a function or class definition) to enforce consistent type usage without knowing beforehand what that type will be.
For example, a simple generic function in TypeScript might look like this:
typescriptfunction identity<T>(arg: T): T { return arg; }
typescriptfunction identity<T>(arg: T): T { return arg; }
In this deliberately simple example, T
is a type variable that will be determined when the function is called. You could call identity
with a number, string, or any other type, and the function would return a value of that same type, ensuring type safety throughout the operation.
Comparing generics with the any
type reveals their true strength. While the any
type allows for any kind of value and effectively opts out of type checking, it comes at the cost of losing type information. Generics, on the other hand, provide a way to keep type information intact, working with the compiler to preserve type safety and provide IntelliSense and code completion features that developers have come to expect in modern IDEs. They are particularly useful when working with collections, algorithms, and data structures, as they allow you to write code that can work with any type without sacrificing type information.

Practical applications of generics
Generics provide a versatile and type-safe way to handle data structures and algorithms in TypeScript. By embracing their power, developers can ensure that their code can work with any type without sacrificing type information. Let's explore some of the practical applications of generics in TypeScript projects.
Using generics with functions
One of the most common use cases for generics is in function creation. By using generics, we can write functions that accept arguments of any type and return values of that same type, ensuring consistency and type safety. Here's an example of a generic function that returns the first item in an array of any type:
typescriptfunction getFirstItem<T>(items: T[]): T | undefined { return items[0]; }
typescriptfunction getFirstItem<T>(items: T[]): T | undefined { return items[0]; }
In the above function, the type variable T
represents the array element type, allowing the function to be used with arrays of numbers, strings, or even complex objects, all while retaining the type information.
Applying generics with interfaces and classes
Generics are also incredibly useful when defining interfaces or classes that operate on a specific type. For instance, consider a generic Queue
class that can work with any type of element:
typescriptclass Queue<T> { private data: T[] = []; push(item: T) { this.data.push(item); } // The returned type is inferred as "T | undefined" from the return statement pop() { return this.data.shift(); } }
typescriptclass Queue<T> { private data: T[] = []; push(item: T) { this.data.push(item); } // The returned type is inferred as "T | undefined" from the return statement pop() { return this.data.shift(); } }
The Queue
class uses the generic type T
for its operations, making the class reusable for any type of data you might need to queue.
Real-world examples of generics in popular libraries/frameworks
Generics are not just a theoretical concept; they're widely used in real-world libraries and frameworks to provide flexible and type-safe solutions. For example, in Angular, you can use generics to define an observable that works with a specific data type:
typescriptimport { Observable } from "rxjs"; function getData<T>(): Observable<T> { // Implementation that returns an observable of type T }
typescriptimport { Observable } from "rxjs"; function getData<T>(): Observable<T> { // Implementation that returns an observable of type T }
In a React context with TypeScript, you might use generics to type built-in hooks or component props:
typescriptinterface Props<T> { items: T[]; renderItem: (item: T) => React.ReactNode; } function List<T>(props: Props<T>) { return ( <div> {props.items.map(props.renderItem)} </div> ); }
typescriptinterface Props<T> { items: T[]; renderItem: (item: T) => React.ReactNode; } function List<T>(props: Props<T>) { return ( <div> {props.items.map(props.renderItem)} </div> ); }
By using generics in functions, classes, interfaces, and even within frameworks, developers can write more maintainable, flexible, and robust code that adapts to a wide range of scenarios. Having explored these practical applications, we'll look deeper into advanced generic techniques that TypeScript offers to help us tackle even more complex design patterns.

Advanced generics techniques
As TypeScript developers become more comfortable with basic generics, they can leverage advanced techniques to build even more powerful and flexible abstractions. Let's look at some of these techniques, including constraints, utility types, and the use of the keyof
keyword.
Constraints in generics
Generics can be further refined by adding constraints that restrict the types that can be used with them. This feature ensures that the generic type adheres to a specific structure or set of properties. Here's an example of a constraint that requires the type to have a length
property:
typescriptfunction logLength<T extends { length: number }>(arg: T): void { console.log(arg.length); }
typescriptfunction logLength<T extends { length: number }>(arg: T): void { console.log(arg.length); }
In this function, the type T
is constrained to types that have a length
property of type number
, such as arrays or strings. This way, the function can be used with confidence that the property will exist on the argument passed to it.
Using keyof with generics
The keyof
operator in TypeScript is used in conjunction with generics to ensure the type safety of property names. It generates a union of known, public property names of a type. Here's how it might be used with generics:
typescriptfunction getProperty<T, K extends keyof T>(obj: T, key: K): T[K] { return obj[key]; }
typescriptfunction getProperty<T, K extends keyof T>(obj: T, key: K): T[K] { return obj[key]; }
When this function is used, TypeScript ensures that the key passed to it actually exists on the object, avoiding runtime errors associated with accessing non-existent properties.
Generic utility types
TypeScript includes a suite of utility types that make it easier to transform types in various ways using generics. Some useful generic utility types are:
Partial<T>
- Makes all properties ofT
optional.Readonly<T>
- Makes all properties ofT
read-only.Pick<T, K>
- Creates a type with a subset of propertiesK
from another typeT
.Record<K, T>
- Creates a type with a set of propertiesK
of typeT
.
These utility types can greatly simplify common type transformations and ensure that your code is concise and expressive. For instance, you can create a type representing a writable version of a read-only interface:
typescriptinterface ReadOnlyPerson { readonly name: string; readonly age: number; } type WritablePerson = Partial<ReadOnlyPerson>;
typescriptinterface ReadOnlyPerson { readonly name: string; readonly age: number; } type WritablePerson = Partial<ReadOnlyPerson>;
With this foundation, the final section will discuss the best practices and common pitfalls when using generics.

Best practices and common pitfalls when using generic types
As developers integrate generics into their TypeScript projects, it's important to follow some best practices to maintain clarity and prevent common pitfalls. In this section, we'll go over essential tips for working with generics and how to avoid mistakes that could lead to complex bugs or decreased code readability.
Best practices for naming generic type variables
The naming of generic type variables should be intuitive and, if possible, descriptive. While single-letter names like T
for "type" are common, sometimes opting for more descriptive names can significantly improve code readability, especially when dealing with multiple generics. For example:
typescriptinterface KeyValuePair<KeyType, ValueType> { key: KeyType; value: ValueType; }
typescriptinterface KeyValuePair<KeyType, ValueType> { key: KeyType; value: ValueType; }
This notation is clearer than simply using K
and V
as the type variables, as it immediately conveys the meaning of each type within the context of a key-value pair.
Avoiding common mistakes with generics
One common mistake with generics is assuming that a generic type has certain properties or methods without proper constraints. This can lead to runtime errors. Always remember to define appropriate constraints when you expect specific behaviors from a type variable:
typescriptfunction calculateLength<T extends { length: number }>(arg: T): number { return arg.length; // Safe as 'length' is ensured by the constraint }
typescriptfunction calculateLength<T extends { length: number }>(arg: T): number { return arg.length; // Safe as 'length' is ensured by the constraint }
Another issue is overusing generics when they're not necessary. Generics should be used to add meaningful flexibility to your code. If a type's range can be narrowed down to a few specific types, it might be better to use a union type instead.
Performance considerations
Generics usually do not have a direct impact on runtime performance, as TypeScript gets compiled to JavaScript, and type information is erased. However, overly complex typing using generics can affect compile-time performance and lead to slower development iteration cycles. Use generics judiciously, and benchmark your compile times if you suspect they are detrimental to your workflow.
Generics should be employed when they contribute to the reusability and type safety of your code. It's wise to use generics if:
- A function, class, or interface operates on a variety of types.
- You find yourself repeating code for different types.
- You need to maintain type relationships across different properties or functions.
However, avoid falling into "generic for the sake of generic" traps. If your code only works with a specific set of known types, generics might add unnecessary complexity without any practical benefit.

Conclusion
In summary, generics in TypeScript are a powerful feature that, when used effectively, can greatly enhance your code's flexibility, reusability, and safety. By understanding their practical applications, mastering advanced techniques, and adhering to best practices, you will be well-equipped to harness the full potential of generics in your TypeScript projects. Remember to integrate them thoughtfully into your development process, and enjoy the type-safe and maintainable code that results from their proper usage.