TypeScript Advanced Types: Working with Conditional Types
Updated on · 6 min read|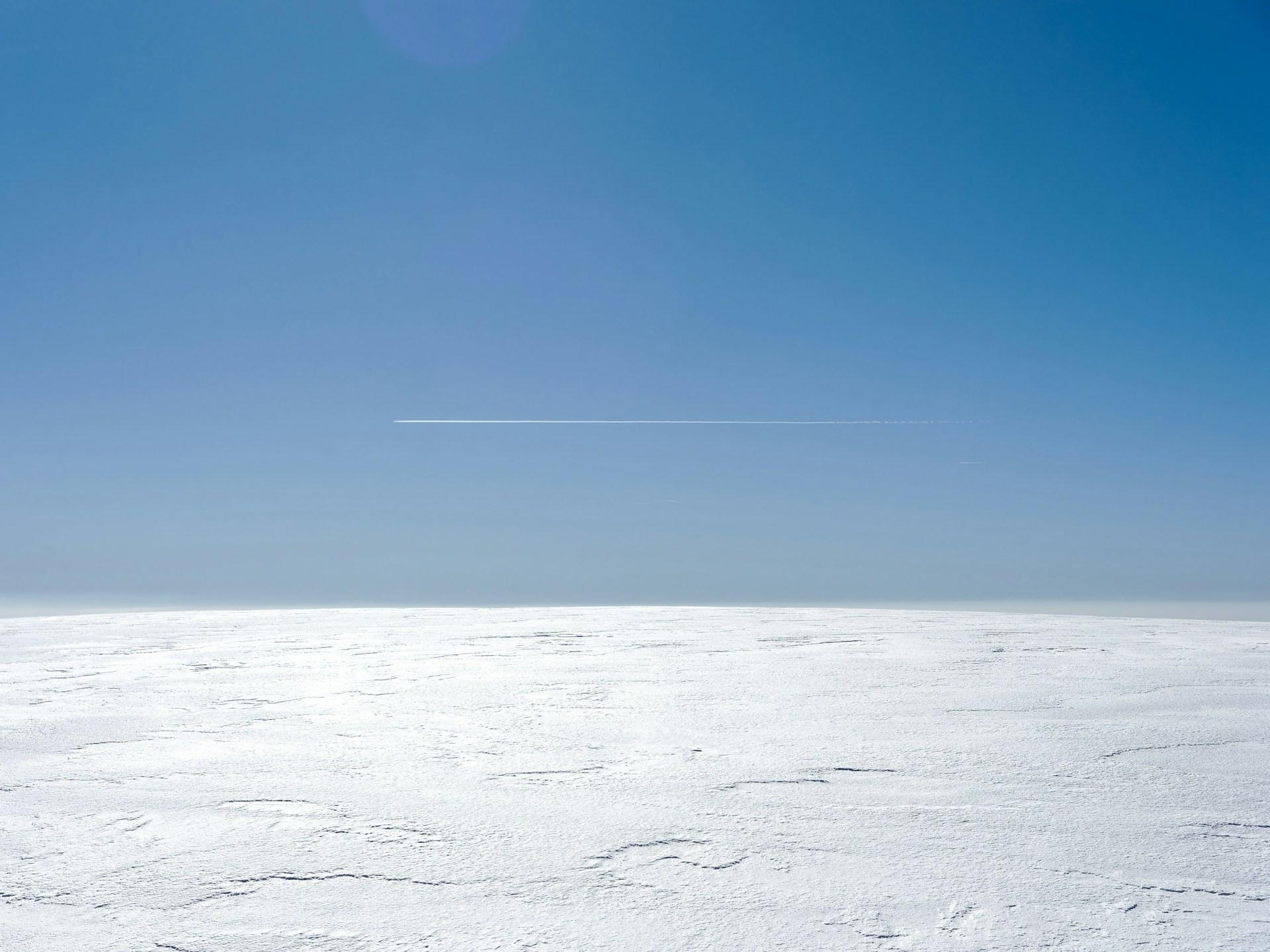
When it comes to building large-scale applications, TypeScript has become an indispensable tool for developers around the world. As a typed superset of JavaScript, it provides the much-needed safety net that helps catch errors early in the development process. By offering a robust type system, TypeScript ensures that the code behaves as expected, reducing the chances of runtime bugs.
Among TypeScript's advanced type features, like generic types, type guards, template literal types and mapped types, conditional types stand out as a powerful tool that brings a level of logic to type definitions, allowing developers to write more flexible and maintainable code. conditional types can be thought of as TypeScript's way of providing 'if-else' logic to types, allowing for type transformations based on certain conditions.
In this blog post, we'll explore how conditional types work, where they are most effective, and some of the advanced patterns they enable. We'll also discuss best practices for using conditional types and consider their limitations, helping you to integrate them into your TypeScript projects with confidence.
Understanding conditional types
Conditional types in TypeScript are a sophisticated feature that allows types to be chosen based on conditions. This concept is similar to conditional expressions (if-else
) in JavaScript, where the outcome depends on a boolean expression. However, in the case of TypeScript, the conditions are based on type relationships rather than actual runtime values.
Syntax and structure
At its core, a conditional type takes a form that looks like this:
typescripttype ConditionalType = SomeType extends OtherType ? TrueType : FalseType;
typescripttype ConditionalType = SomeType extends OtherType ? TrueType : FalseType;
Here's what's happening in this syntax:
SomeType extends OtherType
checks ifSomeType
is assignable toOtherType
.TrueType
is the type that will be used if the condition is true.FalseType
is the type that will be used if the condition is false.
Basic Usage Scenarios
Conditional Types are particularly useful when you are creating utility types, working with generic types, or dealing with situations where the type may vary based on certain conditions.
For instance, you might have a situation where you want a type to resolve differently based on whether the input is an array or not:
typescripttype WrappedValue<T> = T extends any[] ? T[number] : T; // Resolves to string type StringOrStringArray = WrappedValue<string[]>;
typescripttype WrappedValue<T> = T extends any[] ? T[number] : T; // Resolves to string type StringOrStringArray = WrappedValue<string[]>;
Here, WrappedValue
will extract the type of a value wrapped in an array. If T
is not an array, it just returns T
itself.
By using conditional types, TypeScript developers can create sophisticated type definitions that respond dynamically to the types that they're applied to — promoting reusability, enhancing type safety, and paving the way for more expressive code.

Advanced use cases and patterns
As developers become more familiar with conditional types, they can leverage them for more advanced scenarios that require a nuanced approach to type manipulation and inference. Here, we'll explore how to use conditional types to create intelligent types that respond to the shape of the data they represent.
Leveraging conditional types for type inference
One of the most powerful use cases for conditional types is in type inference within function signatures. By using the infer
keyword, TypeScript can infer types in the context of the condition. This is particularly useful in higher-order functions and type manipulation utilities.
For example, extracting the return type of a function:
typescripttype ReturnType<T> = T extends (...args: any[]) => infer R ? R : never; // Using ReturnType function getString(): string { return "Hello, TypeScript!"; } // Resolves to string type StringReturnType = ReturnType<typeof getString>;
typescripttype ReturnType<T> = T extends (...args: any[]) => infer R ? R : never; // Using ReturnType function getString(): string { return "Hello, TypeScript!"; } // Resolves to string type StringReturnType = ReturnType<typeof getString>;
In this snippet, ReturnType
conditionally checks if T
is a function, and if so, it uses the infer
keyword to capture the return type of that function (R
) and uses it in the true branch of the conditional. If T
is not a function type, it resolves to never
.
Filtering types with utility types
Conditional types also empower developers to create utility types that can filter or modify types based on certain criteria. Two built-in utility types that showcase this use case are Exclude
and Extract
.
typescript// Exclude from T those types that are assignable to U type Exclude<T, U> = T extends U ? never : T; // Extract from T those types that are assignable to U type Extract<T, U> = T extends U ? T : never;
typescript// Exclude from T those types that are assignable to U type Exclude<T, U> = T extends U ? never : T; // Extract from T those types that are assignable to U type Extract<T, U> = T extends U ? T : never;
These utilities are particularly valuable when you want to create types that exclude or include specific members of union types:
typescript// Given these types type SomeTypes = string | number | boolean; type StringOrNumber = string | number; // Exclude boolean from SomeTypes type NoBoolean = Exclude<SomeTypes, boolean>; // string | number // Extract only the types in SomeTypes that are also in StringOrNumber type StringsAndNumbers = Extract<SomeTypes, StringOrNumber>; // string | number
typescript// Given these types type SomeTypes = string | number | boolean; type StringOrNumber = string | number; // Exclude boolean from SomeTypes type NoBoolean = Exclude<SomeTypes, boolean>; // string | number // Extract only the types in SomeTypes that are also in StringOrNumber type StringsAndNumbers = Extract<SomeTypes, StringOrNumber>; // string | number
Building type-safe api handlers
API handlers can benefit greatly from conditional types, especially when you want to ensure that the response type matches the expected payload for different endpoints.
Imagine creating a type-safe API handler function where the return type changes based on a passed parameter:
typescripttype ApiResponse<T> = T extends "/user" ? { user: string } : T extends "/settings" ? { settings: string } : never; function getApiEndpoint<T extends string>(path: T): ApiResponse<T> { // Mock API call return {} as ApiResponse<T>; } // The return type will be { user: string } const userResponse = getApiEndpoint("/user"); // The return type will be { settings: string } const settingsResponse = getApiEndpoint("/settings");
typescripttype ApiResponse<T> = T extends "/user" ? { user: string } : T extends "/settings" ? { settings: string } : never; function getApiEndpoint<T extends string>(path: T): ApiResponse<T> { // Mock API call return {} as ApiResponse<T>; } // The return type will be { user: string } const userResponse = getApiEndpoint("/user"); // The return type will be { settings: string } const settingsResponse = getApiEndpoint("/settings");
In this example, ApiResponse
is a conditional type that selects the appropriate response type depending on the endpoint string passed to the function, thereby enforcing type safety at the compiler level, even before the actual API call is made.
Defensive programming with conditional types
Defensive programming aims to make software more robust by anticipating potential problems. TypeScript's conditional types play a crucial role in this by enabling type-based validations. For example:
typescripttype SafeInput<T> = T extends string | number ? T : never; function handleInput<T>(input: T): SafeInput<T> { if (typeof input !== "string" && typeof input !== "number") { throw new Error("Invalid input type"); } return input; // processing is safe here }
typescripttype SafeInput<T> = T extends string | number ? T : never; function handleInput<T>(input: T): SafeInput<T> { if (typeof input !== "string" && typeof input !== "number") { throw new Error("Invalid input type"); } return input; // processing is safe here }
In handleInput
, the SafeInput
type enforces that only strings or numbers are processed. Anything else triggers an error, ensuring only valid code paths are executed.
This technique of using conditional types is particularly valuable when working with forms or other user inputs and their validation, where you want to ensure that the data you're working with is of the expected type.
Conditional types in these scenarios enforce a direct relationship between input values and output types, which is a potent way to reduce the risk of mismatched data types and logical errors in handling dynamic and polymorphic structures in your codebase.

Best practices and limitations
Using conditional types effectively requires an understanding of best practices and an awareness of their limitations. Knowing how to apply conditional types pragmatically can greatly improve the maintainability and readability of your TypeScript code.
Tips for effective usage
Here are some tips for making the most out of conditional types in TypeScript projects:
- Keep conditions simple: complex conditions in conditional types can quickly become hard to read and maintain. Strive to keep your type conditions as straightforward as possible.
- Use type aliases: long conditional types can be broken into smaller, named types using type aliases. This can help with readability and reusability.
- Leverage utility types: TypeScript comes with built-in utility types that use conditional types under the hood. Familiarize yourself with these utilities as they can often save you from reinventing the wheel.
- Test your types: as with any part of a codebase, testing is vital. Consider using tools that support testing TypeScript types, such as
dtslint
ortsd
.
Potential pitfalls to avoid
While conditional types are a powerful tool, they can introduce complexity that may trip you up if you're not careful:
- Avoid excessive nesting: nested conditional types can be hard to untangle and understand, which can lead to errors that are difficult to diagnose.
- Watch out for circular references: it's possible to create inadvertently circular references with conditional types, which can result in hard-to-debug compiler errors.
Limitations and when not to use conditional types
Despite their versatility, conditional types have some limitations:
- Compile-time, not runtime: conditional types operate at the type level and cannot be used to make runtime decisions in your JavaScript code. Misunderstanding this can lead to incorrect assumptions about code behavior.
- Not for every use case: while they can be used to solve a wide range of problems, conditional types are not always the right tool. Avoid using them for simple types or when a regular interface or type would suffice, as this complication can limit code clarity.
Understanding when and when not to use conditional types is a key part of mastering TypeScript. They are best reserved for situations where type flexibility needs to closely reflect complex or variable structures within your code.

Conclusion
Conditional types in TypeScript are a profound extension of the language's type system, providing a way to apply logical conditions to type behavior. They empower developers to write more expressive, flexible, and maintainable type definitions, paving the way for sophisticated type transformations and increased safety in handling complex data structures.
Throughout this post, we've explored the essentials of conditional types, dived into their advanced applications, and provided practical advice for their use.
References and resources
- Beyond Console.log: Debugging Techniques In JavaScript
- Error Handling and Defensive Programming with TypeScript
- Form Validation with React Hook Form
- Managing Forms with React Hook Form
- TypeScript Template Literal Types: Practical Use-Cases for Improved Code Quality
- TypeScript documentation
- Understanding and Implementing Type Guards In TypeScript
- Unlocking the Power of TypeScript's Mapped Types
- Using Generics In TypeScript: A Practical Guide
- Working with Union Types In TypeScript