Unlocking the Power of TypeScript's Mapped Types
Updated on · 6 min read|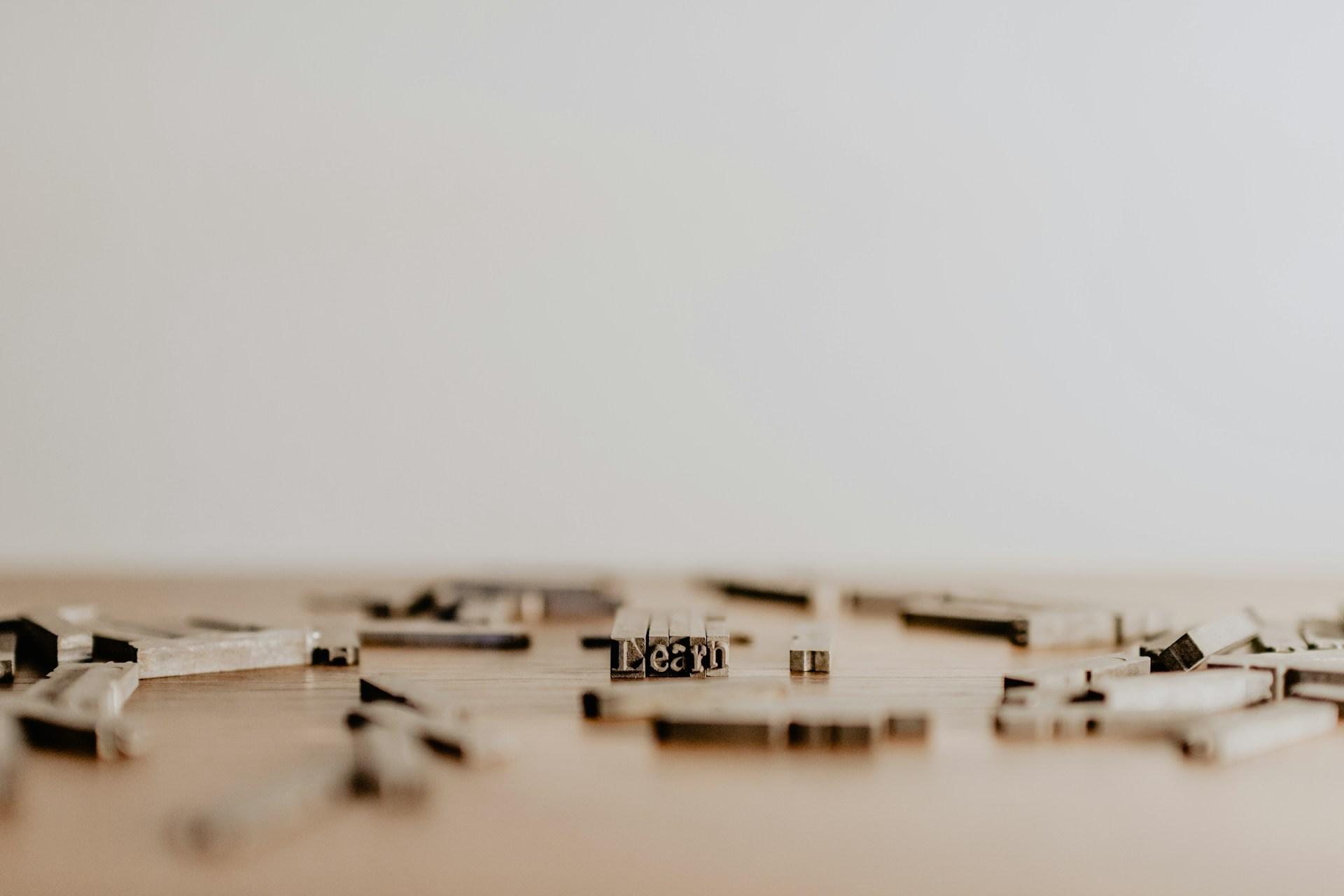
TypeScript, as an extension of JavaScript, brings static typing to the table, allowing developers to catch errors early in the development process and manage codebases with greater confidence. Among TypeScript's advanced type features, like generic types, type guards and template literal types, mapped types stand out as a powerful tool for creating new types based on existing ones. Mapped types enable programmers to iterate through the keys of other types and create transformed versions of those types in a succinct, maintainable way. This ability enhances code reusability and helps in managing complex type transformations effortlessly.
This post aims to explore the capabilities provided by TypeScript’s mapped types. We’ll start by looking at the core concepts that underpin mapped types, then progress to exploring a range of practical applications that demonstrate their versatility. Reading this, developers new to mapped types will gain a foundational understanding, while seasoned TypeScript users may uncover new insights to improve their type manipulation techniques.
Understanding the basics of mapped types
Mapped types in TypeScript are akin to a transformative tool that lets us create new types by transforming each property in an existing type one by one. They follow a syntax that might look complex at first glance, but becomes clear once broken down:
typescripttype MappedType<T> = { [P in keyof T]: T[P]; };
typescripttype MappedType<T> = { [P in keyof T]: T[P]; };
In this structure, T
represents a generic type that we will be operating on, while P
stands for the properties of T
. The keyof
keyword produces a union of known, public property names of T
, while the in
keyword iterates over those property names. What follows after the colon character (:
) is the type specification for the properties in the newly created type.
Mapped types are related to index signatures, which describe the ability to access object properties via index keys. With mapped types, however, we are not just setting the stage for dynamic property access, but actively creating a new type with properties that have undergone some form of transformation—whether it's changing the property type, making properties optional or readonly, or excluding certain properties altogether.
To illustrate, let’s consider a simple example of a mapped type that takes an existing type and makes all of its properties optional:
typescripttype Partial<T> = { [P in keyof T]?: T[P]; };
typescripttype Partial<T> = { [P in keyof T]?: T[P]; };
If we have an existing type User
with properties name
and age
, applying the Partial
mapped type to it will result in a new type where both name
and age
are optional properties.
Use cases for mapped types
Mapped types' true power is clear when used in complex type transformations. Below are several use cases demonstrating how mapped types can be adopted to solve common and intricate problems in type manipulation.
Creating read-only types
The versatility of mapped types allows us to lock down the mutability of properties. This is particularly useful when working with immutable data. Consider the Readonly
mapped type:
typescripttype Readonly<T> = { readonly [P in keyof T]: T[P]; };
typescripttype Readonly<T> = { readonly [P in keyof T]: T[P]; };
These predefined utilities in TypeScript make every property in T
readonly.
Property selection and omission
We can selectively pick or omit properties from types by using utilities such as Pick
and Omit
, which can be defined as:
typescripttype Pick<T, K extends keyof T> = { [P in K]: T[P]; }; type Omit<T, K extends keyof T> = Pick<T, Exclude<keyof T, K>>;
typescripttype Pick<T, K extends keyof T> = { [P in K]: T[P]; }; type Omit<T, K extends keyof T> = Pick<T, Exclude<keyof T, K>>;
Pick
creates a new type by picking a set of properties K
from type T
, while Omit
creates a type by excluding a set of properties K
from T
.
Conditional type transformations with mapped types
Mapped types can also be used in tandem with conditional types and infer keyword to transform an existing type based on certain conditions. This enables more complex logic to be applied during the mapping process. An example of this is transforming an API response type based on the properties of the original type:
typescripttype ApiResponse<T> = { [P in keyof T]: T[P] extends { type: infer Type; required: true } ? { value: Type; error: string } : T[P] extends { type: infer Type; required: boolean } ? { value?: Type; error?: string } : never; // You may want to handle unexpected shapes differently }; interface UserRequest { username: { type: string; required: true }; age: { type: number; required: false }; } // ApiResponse<UserRequest> is: // { // username: { value: string; error: string }; // age: { value?: number; error?: string }; // }
typescripttype ApiResponse<T> = { [P in keyof T]: T[P] extends { type: infer Type; required: true } ? { value: Type; error: string } : T[P] extends { type: infer Type; required: boolean } ? { value?: Type; error?: string } : never; // You may want to handle unexpected shapes differently }; interface UserRequest { username: { type: string; required: true }; age: { type: number; required: false }; } // ApiResponse<UserRequest> is: // { // username: { value: string; error: string }; // age: { value?: number; error?: string }; // }
In this example, the ApiResponse
mapped type transforms the UserRequest
type based on the properties of the original type. This is a powerful way to create types that are contextually dependent on the shape of other types.
Dynamic property names with template literals
Mapped types combined with template literal types enable typings for dynamic property names based on certain patterns. This feature is particularly useful for defining property names based on external conditions, such as API response keys:
typescripttype ResponseKeys = "success" | "error"; type APIResponses = { [K in ResponseKeys as `${K}Response`]: K extends "success" ? { data: unknown } : { error: string }; }; // APIResponses is: // { // successResponse: { data: unknown }; // errorResponse: { error: string }; // }
typescripttype ResponseKeys = "success" | "error"; type APIResponses = { [K in ResponseKeys as `${K}Response`]: K extends "success" ? { data: unknown } : { error: string }; }; // APIResponses is: // { // successResponse: { data: unknown }; // errorResponse: { error: string }; // }
Through this advanced utility, we can ensure that our type system remains flexible and robust, capable of accurately reflecting dynamically shaped JavaScript objects.
Mapped types with form events
When working with the types for form events in React, it is common to deal with a variety of input types and event handlers. For example, you may need to handle text changes, selections, and checks. Mapped types allow you to create a generic handler type that maps event types to their specific Event
object, improving type safety and reducing the chances of errors.
typescripttype FormEventMap = { text: React.ChangeEvent<HTMLInputElement>; select: React.ChangeEvent<HTMLSelectElement>; checkbox: React.ChangeEvent<HTMLInputElement>; }; type FormEventHandler<T extends keyof FormEventMap> = ( event: FormEventMap[T], ) => void; // Usage const handleTextChange: FormEventHandler<"text"> = (event) => { // event is correctly typed as ChangeEvent<HTMLInputElement> console.log(event.currentTarget.value); }; const handleCheckboxChange: FormEventHandler<"checkbox"> = (event) => { // event is correctly typed as ChangeEvent<HTMLInputElement> console.log(event.currentTarget.checked); };
typescripttype FormEventMap = { text: React.ChangeEvent<HTMLInputElement>; select: React.ChangeEvent<HTMLSelectElement>; checkbox: React.ChangeEvent<HTMLInputElement>; }; type FormEventHandler<T extends keyof FormEventMap> = ( event: FormEventMap[T], ) => void; // Usage const handleTextChange: FormEventHandler<"text"> = (event) => { // event is correctly typed as ChangeEvent<HTMLInputElement> console.log(event.currentTarget.value); }; const handleCheckboxChange: FormEventHandler<"checkbox"> = (event) => { // event is correctly typed as ChangeEvent<HTMLInputElement> console.log(event.currentTarget.checked); };
By using mapped types to map input types to their correct event types, we can define event handlers that are contextually appropriate, resulting in clearer and safer code.
These use cases illustrate that mapped types are indispensable tools in the TypeScript toolkit. They encourage code safety, flexibility, and reusability when working with complex data structures and APIs. The next section will provide guidelines on using mapped types effectively and responsibly in various contexts.
Best practices for working with mapped types
Using mapped types effectively involves understanding both their strengths and limitations. By adhering to best practices, developers can ensure they are leveraging mapped types to their fullest potential without introducing unnecessary complexity or performance overheads. Here are some tips and considerations for working with mapped types in TypeScript.
When to use mapped types
Mapped types are most beneficial when you need to create types that are variations of existing types, especially when dealing with many related types that only differ in certain aspects, like optionality, mutability, or subsets of properties. They are also useful for creating utility types that can handle a variety of similar transformations across different types in your codebase.
Balancing type safety and complexity
While mapped types can precisely describe the shape of your data, they can also introduce complexity that might make your types harder to read or maintain. Strike a balance by using mapped types when they enhance readability and maintainability - for example, when they help avoid repetition or when they clearly convey the intent of a type transformation. Avoid overusing mapped types for simple structures where a basic type alias or interface would suffice.
Documentation and code clarity
Given their sometimes complex syntax, mapped types should be accompanied by comments or documentation that explain their purpose and usage within the code. This practice is especially important when defining custom utility types that perform non-trivial transformations. Good documentation ensures that team members understand the logic behind these types and can use them correctly.
Type aliases for common mappings
If you find yourself repeatedly using the same mapped type pattern, consider abstracting it into a reusable type alias. This not only reduces repetition but also makes your code cleaner and easier to refactor in the future.
Leveraging TypeScript's utility types
Familiarize yourself with TypeScript's built-in utility types such as Partial
, Readonly
, Pick
, and Record
. These cover many common scenarios where mapped types are useful, and leveraging them can save you time and effort compared to writing custom mapped types from scratch.
By applying these best practices, you can integrate mapped types into your TypeScript workflow in a way that enhances type safety and developer experience, without compromising on code legibility or performance. Mapped types are a means to an end - a way to model a domain more accurately in TypeScript - and should be used judiciously to align with any project's needs.
Conclusion
In conclusion, TypeScript's mapped types are a powerful feature that can transform and control types with precision and ease. They bring an unmatched level of flexibility to the static type system, allowing for expressive and maintainable code. Balancing their advanced capabilities with careful consideration regarding their appropriate use will ensure that your type definitions remain accessible and efficient. With practice and thoughtful application, mapped types will undoubtedly become a valuable tool in any developer's TypeScript arsenal.
References and resources
- Immutable Array Operations In JavaScript: Introducing ToSorted, ToSpliced, and ToReversed
- TypeScript Advanced Types: Working with Conditional Types
- TypeScript Template Literal Types: Practical Use-Cases for Improved Code Quality
- TypeScript website
- TypeScript's Infer Keyword: Unlocking Type Information
- TypeScript: Typing Form Events In React
- Understanding and Implementing Type Guards In TypeScript
- Using Generics In TypeScript: A Practical Guide