TypeScript's Infer Keyword: Unlocking Type Information
Updated on · 7 min read|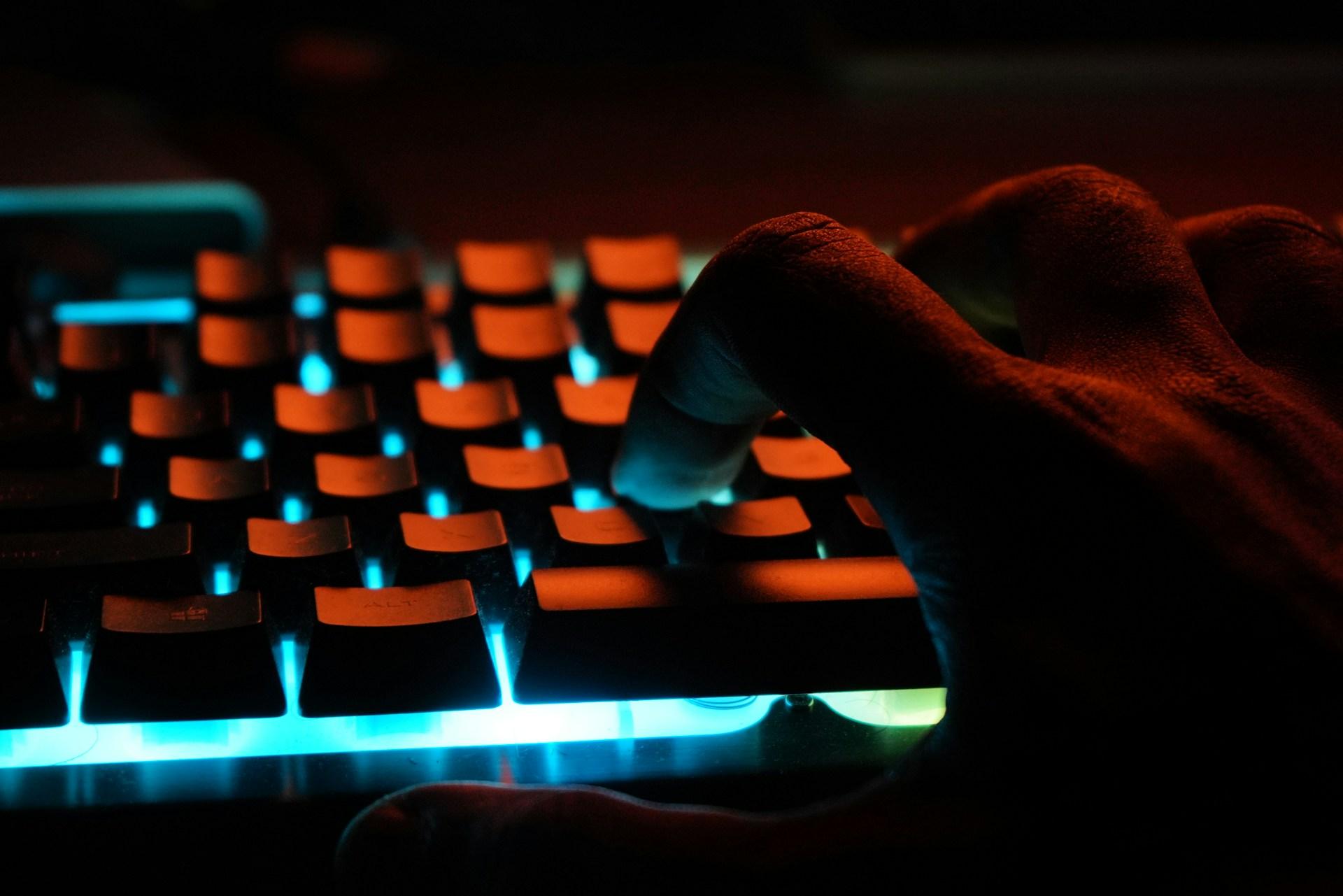
TypeScript's infer
keyword is a powerful tool for extracting type information from other types. Introduced as a part of TypeScript's advanced types, such as generic types, type guards, mapped types and template literal types, infer
plays a crucial role in the static type system. It provides the flexibility needed to extract types in a way that encourages code reusability and maintainability while upholding strong type safety. Understanding and applying the infer
keyword can lead to more expressive and scalable type declarations.
Understanding type inference in TypeScript
Given that type inference has been extensively covered in another article, this post will offer only a brief overview of the concept.
What is type inference?
Type inference is a cornerstone of TypeScript's functionality. It is the mechanism through which the compiler deduces the types of variables, functions, and properties when developers do not annotate them explicitly. By examining the initializations and usage patterns, TypeScript can infer type information, thereby reducing the verbosity of code and streamlining development.
For example, when a variable is assigned a number, TypeScript assumes the type to be number
, thus freeing the developer from having to annotate it manually:
typescriptlet inferredNumber = 42; // TypeScript infers the type as number
typescriptlet inferredNumber = 42; // TypeScript infers the type as number
Role of the infer keyword
While the general concept of type inference relates to TypeScript’s automatic detection of types, the infer
keyword takes this a step further. Within TypeScript's type system, infer
is used to introduce a type variable inside type expressions. This newly introduced type variable can then be used to capture and use types dynamically within other types, such as in conditional types or type manipulation utilities.
For example, the infer
keyword in TypeScript enhances type manipulation by allowing for the extraction and conditional application of types within generic operations, simplifying complex type definitions, enabling precise type extraction from arrays, functions, and promises, and facilitating the creation of highly reusable and adaptable type utilities, ultimately improving code maintainability and safety across diverse use cases.
Practical applications of infer
The infer
keyword finds application in a variety of scenarios, each of which demonstrates its versatility and power. The next section will explore some of these practical applications, showcasing how infer
can be used to create more flexible and reusable type definitions.
Inferring types within conditional types
The use of infer
within conditional types is particularly powerful as it allows TypeScript to extract type information conditionally. Conditional types enable developers to write types that behave differently based on the input types they are provided. With infer
, these types can become more expressive and dynamic, facilitating the creation of type utilities that can adapt to a variety of scenarios.
For example, one might write a type that extracts the element type of an array:
typescripttype ElementType<T> = T extends (infer U)[] ? U : T; type Result = ElementType<number[]>; // number
typescripttype ElementType<T> = T extends (infer U)[] ? U : T; type Result = ElementType<number[]>; // number
The ElementType
type here will extract the type U
if T
is an array type. Otherwise, it will simply return T
itself. This is useful when handling operations on arrays where the element's type needs to be known. The infer
keyword allows the type to adapt to the input type, making it more reusable and flexible.
Extracting return types from functions
Another practical use of the infer
keyword is extracting the return type of a function. This is commonly done when interfacing with functions whose implementations might change, thus keeping dependent types in sync with these changes.
A type to extract a function’s return type might look like the following:
typescripttype ReturnType<T> = T extends (...args: any[]) => infer R ? R : any; function greet(name: string) { return `Hello, ${name}!`; } type GreetReturnType = ReturnType<typeof greet>; // string
typescripttype ReturnType<T> = T extends (...args: any[]) => infer R ? R : any; function greet(name: string) { return `Hello, ${name}!`; } type GreetReturnType = ReturnType<typeof greet>; // string
With this ReturnType
utility type, it becomes trivial to capture the return type of a function for reuse in other parts of the application without having to duplicate the definition. TypeScript's built-in ReturnType
utility type works similarly to the custom ReturnType
type defined above.
Capturing type information for higher-order functions
Higher-order functions, which are functions that take other functions as arguments or return a function, can benefit greatly from the infer
keyword. When defining types for higher-order functions, it's often necessary to capture and work with the types of arguments and return values of the functions being passed around.
For example, let's look at function composers or pipelines, which require knowing the return type of one function to match the parameter type of the next. With the infer
keyword, the types can flow through the composition unimpeded:
typescripttype NumberToString = (a: number) => string; type ComposedFunction< FirstFunction extends Function, SecondFunction extends Function, > = FirstFunction extends (arg: infer FirstArg) => any ? SecondFunction extends (arg: ReturnType<FirstFunction>) => any ? (arg: FirstArg) => ReturnType<SecondFunction> : never : never; const numberToString: NumberToString = (num) => `Number is ${num}`; const stringToLength: (str: string) => number = (str) => str.length; const composed: ComposedFunction< typeof numberToString, typeof stringToLength > = (num) => stringToLength(numberToString(num)); const length = composed(5); // Length would be the string length of "Number is 5"
typescripttype NumberToString = (a: number) => string; type ComposedFunction< FirstFunction extends Function, SecondFunction extends Function, > = FirstFunction extends (arg: infer FirstArg) => any ? SecondFunction extends (arg: ReturnType<FirstFunction>) => any ? (arg: FirstArg) => ReturnType<SecondFunction> : never : never; const numberToString: NumberToString = (num) => `Number is ${num}`; const stringToLength: (str: string) => number = (str) => str.length; const composed: ComposedFunction< typeof numberToString, typeof stringToLength > = (num) => stringToLength(numberToString(num)); const length = composed(5); // Length would be the string length of "Number is 5"
Here, we define a Compose
type that ensures the output type of the first function (Fn
) matches the expected input type of the second function argument, promoting type safety throughout the composition process.
Working with complex type manipulations
Beyond the basic cases, infer
finds application in more complex type manipulations. For example, in the creation of types that unwrap Promise types or to handle higher-order functions where multiple layers of function types may occur, infer
can be used to drill down and capture the underlying types needed.
Consider the unwrapping of a promise type:
typescripttype UnwrapPromise<T> = T extends Promise<infer U> ? U : T; const promise: Promise<string> = Promise.resolve("Hello, world!"); type Unwrapped = UnwrapPromise<typeof promise>; // string const nonPromise: string = "Hello, world!"; type UnwrappedNonPromise = UnwrapPromise<typeof nonPromise>; // string
typescripttype UnwrapPromise<T> = T extends Promise<infer U> ? U : T; const promise: Promise<string> = Promise.resolve("Hello, world!"); type Unwrapped = UnwrapPromise<typeof promise>; // string const nonPromise: string = "Hello, world!"; type UnwrappedNonPromise = UnwrapPromise<typeof nonPromise>; // string
This type will correctly extract the type U
if T
is a promise resolving to U
. Such utility types can vastly simplify asynchronous handling by providing clearer type information about the resolved values.
It should be noted that in the non-promise example, we need to explicitly specify the type of nonPromise
variable as string
when using a const
declaration. Otherwise, TypeScript will infer the type of nonPromise
as the literal type "Hello, world!"
, instead of string
. Alternatively, using let
instead of const
to declare nonPromise
could allow TypeScript to infer a more general string
type.
Whether it is working with nested array types, function compositions, or promises, infer
provides a robust way to capture and propagate type information, thus unlocking sophisticated type transformations and enhancing code safeness and maintainability.
Best practices for using the infer keyword
The infer
keyword is a powerful tool for creating expressive and flexible type definitions in TypeScript. However, it is essential to use it judiciously and in a way that aligns with best practices for writing maintainable and scalable TypeScript code. The following best practices can help developers make the most of the infer
keyword.
Avoiding overuse and complexity
While infer
is undoubtedly powerful, it is imperative to use it judiciously. Overusing the infer
keyword can lead to excessively complex types which become challenging to understand and maintain. Type definitions should remain as simple as possible without compromising the integrity of the type information they represent.
Developers should strive to create type abstractions that do not obscure the underlying types to the point where the logic is no longer transparent. Code reviews and pair programming can be beneficial practices to ensure that the use of the infer
keyword enhances rather than obfuscates the code.
Simplify nested types extraction
Nested type extractions can get messy and hard to read. Using infer
, you can simplify the process, making your code cleaner and more straightforward. Instead of creating several levels of type mappings and lookups, use infer
to succinctly extract the type information you need. This not only improves readability but also reduces the likelihood of errors.
Create reusable type utilities
The infer
keyword shines when used to create reusable type utilities. For instance, utilities for extracting return types of functions, the item types of arrays, or the resolved types of promises can significantly reduce duplication and increase the consistency of your type manipulations. These utilities can then be used across your codebase to infer types dynamically, ensuring that your type definitions are always aligned with your data structures and logic flows.
That being said, TypeScript provides a set of built-in utility types that leverage infer
to provide a wide range of type manipulation capabilities, so it's worth checking if the utility you need is already available before creating a custom one.
Conclusion
This post has highlighted how the infer
keyword in TypeScript can be advantageous for developers looking to harness the full potential of the language's advanced type system. By understanding and applying infer
within conditional types, one can extract and manipulate type information in a more dynamic and reusable way. Whether for extracting function return types, unwrapping promise types, or more complex type manipulations, infer
brings a level of expressiveness to type definitions that helps in maintaining strong type safety without compromising on code clarity.
However, it's crucial to approach infer
with a mindset geared towards simplicity and maintainability. Overuse or inappropriate use can lead to complexity that works against the benefits that TypeScript provides. Striking the right balance is key; it requires careful consideration of when and how to use infer
to enhance the readability, safety, and maintainability of the code.
References and resources
- Higher-Order Functions In JavaScript
- Introduction to Type Inference in TypeScript
- TypeScript Advanced Types: Working with Conditional Types
- TypeScript Template Literal Types: Practical Use-Cases for Improved Code Quality
- TypeScript website
- TypeScript: ReturnType
- TypeScript: Utility types
- Understanding and Implementing Type Guards In TypeScript
- Unlocking the Power of TypeScript's Mapped Types
- Using Generics In TypeScript: A Practical Guide