Introduction to Type Inference In TypeScript
Updated on · 7 min read|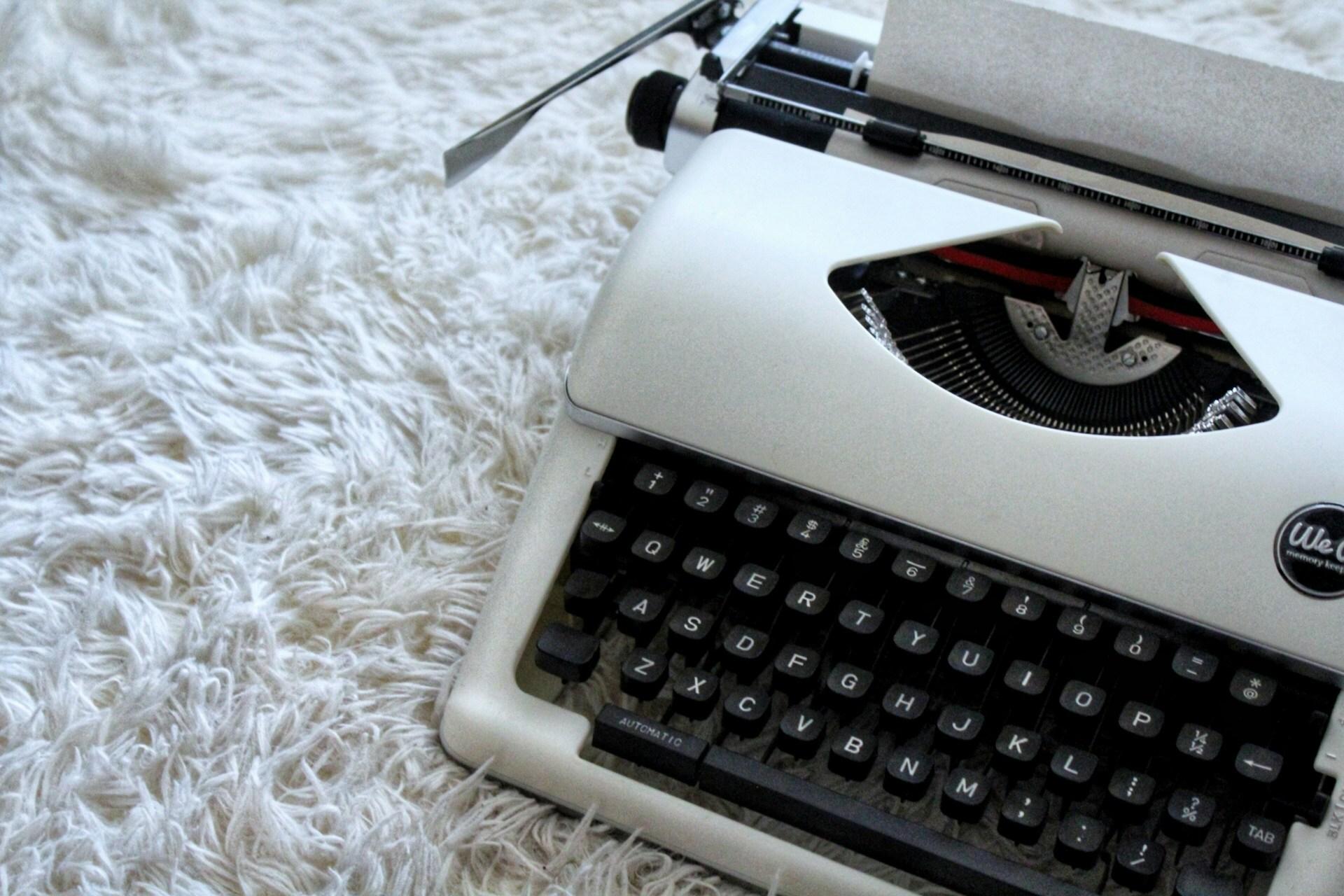
TypeScript has become an increasingly popular choice for developers aiming to bring static typing to JavaScript projects. One of the key features that sets TypeScript apart is its ability to perform type inference. This feature allows developers to write code more quickly without sacrificing the benefits of a strong type system.
Type inference in TypeScript helps in determining variable or expression types based on their value when it's not explicitly specified. When TypeScript can infer the type of a variable, there is no need to annotate the variable with a type, which can make the code cleaner and easier to read.
In cases where type inference is not straightforward, TypeScript provides various ways in which a developer can guide the compiler toward the correct typing. Understanding the power of TypeScript's type inference can lead to more concise and maintainable code. This post will demonstrate how type inference is a key aspect of TypeScript, aiding developers in their projects across various scenarios.
Understanding type inference in TypeScript
Type inference is one of the pillars of TypeScript's functionality, enhancing developers' ability to produce reliable and maintainable software with less verbose and redundant code. It is important to understand how TypeScript's compiler deduces type information when it is not explicitly defined.
The basics of type inference
At its core, type inference is TypeScript's ability to deduce types based on certain rules and the context in which values are used. Here's how it typically works:
- Initialization: when a variable is initialized, TypeScript infers its type based on the value assigned to it. If you assign a string to a variable, TypeScript will automatically infer its type as
string
.
typescriptlet message = "Hello World"; // `message` is inferred to be of type `string`
typescriptlet message = "Hello World"; // `message` is inferred to be of type `string`
- No initialization: if a variable is declared but not initialized, TypeScript infers it has the type
any
, allowing it to hold any value without type-check constraints.
typescriptlet message; // `message` is inferred to be of type `any`
typescriptlet message; // `message` is inferred to be of type `any`
- Best common type: when an array is initialized with various types, TypeScript infers the type based on the best common type algorithm, which looks at all the elements types and selects a compatible type that works for all elements.
typescriptlet collection = [1, 2, "3"]; // `collection` is inferred to be of type `(number | string)[]`
typescriptlet collection = [1, 2, "3"]; // `collection` is inferred to be of type `(number | string)[]`
Inference in functions
Functions are a cornerstone in TypeScript, and inference plays a significant role in their type safety. TypeScript can infer the return type of functions based on the return statements contained within them. If a function returns different types of data, TypeScript will determine the return type based on a union of the types.
typescriptfunction getId(id) { return id.toString(); }
typescriptfunction getId(id) { return id.toString(); }
In the above example, TypeScript infers the return type as string
, even without explicit annotations, because the toString()
method always returns a string.
Although TypeScript typically requires types for function parameters, there are occasions where it can infer parameter types, particularly when a function is used in a specific context or when default parameter values are provided.
typescriptaddEventListener("keydown", (event) => { console.log(event.key); // `event` is inferred as `KeyboardEvent` });
typescriptaddEventListener("keydown", (event) => { console.log(event.key); // `event` is inferred as `KeyboardEvent` });
Here, the type of event
is inferred as KeyboardEvent
because of the context in which the function is used — in this case, as an event handler for keyboard events.
Moreover, when functions have default parameter values, TypeScript uses those values to infer the types of the parameters.
typescriptfunction greeting(name = "Guest") { return `Hello, ${name}`; // `name` is inferred as `string` }
typescriptfunction greeting(name = "Guest") { return `Hello, ${name}`; // `name` is inferred as `string` }
The parameter name
is inferred as string
because the default value assigned is a string.
TypeScript also uses contextual typing to infer parameter types in other scenarios, such as when assigning functions to variables or properties defined with specific types.
typescripttype ClickHandler = (event: MouseEvent) => void; const handleClick: ClickHandler = (event) => { // `event` is inferred to be of type `MouseEvent` console.log(event.clientX, event.clientY); };
typescripttype ClickHandler = (event: MouseEvent) => void; const handleClick: ClickHandler = (event) => { // `event` is inferred to be of type `MouseEvent` console.log(event.clientX, event.clientY); };
In this case, the type of event
in the handleClick
function is inferred to be MouseEvent
, as the function is assigned to a variable with a type that specifies the parameter's type.

Advanced type inference features
TypeScript offers advanced type inference capabilities that provide a deeper understanding and control over how types are handled in various scenarios. These features can significantly benefit developers working on complex applications by reducing code verbosity and improving maintainability.
Type inference with generics
Generics add another layer of flexibility and reusability in TypeScript's type system. TypeScript can infer types within generic functions or classes based on the arguments or instances that are passed, without explicit type annotations. Here is where the magic of type inference truly shines, as it can provide strong typing for complex operations like data transformations or handling collections.
For instance, a generic function that returns the argument it receives can automatically infer the type of the return based on the input:
typescriptfunction wrapInArray<T>(value: T) { return [value]; } let stringArray = wrapInArray("hello"); // `stringArray` is inferred as `string[]` let numberArray = wrapInArray(10); // `numberArray` is inferred as `number[]`
typescriptfunction wrapInArray<T>(value: T) { return [value]; } let stringArray = wrapInArray("hello"); // `stringArray` is inferred as `string[]` let numberArray = wrapInArray(10); // `numberArray` is inferred as `number[]`
In the code snippet above, TypeScript intelligently deduces the generic type T
based on the type of the argument provided to the function call.
Type guards and inference
Type guards are a way to provide hints to the TypeScript compiler about the type of a variable inside a specific code block. Using type guards affects the type inference by narrowing types based on runtime checks.
typescriptfunction isString(value: unknown): value is string { return typeof value === "string"; } // `unknownValue` has a type `unknown` const unknownValue: unknown = getSomeValue(); if (isString(unknownValue)) { // Within this block, `unknownValue` is inferred as `string` console.log(unknownValue.toUpperCase()); }
typescriptfunction isString(value: unknown): value is string { return typeof value === "string"; } // `unknownValue` has a type `unknown` const unknownValue: unknown = getSomeValue(); if (isString(unknownValue)) { // Within this block, `unknownValue` is inferred as `string` console.log(unknownValue.toUpperCase()); }
Proper use of type guards can make code safer and help avoid unnecessary type assertions and excess annotation.
Mapped types and inference
Mapped types, which create new types by transforming existing ones, play well with type inference to create flexible and reusable type definitions. However, developers should be cautious when creating complex mapped types as excessive or improper use can lead to confusing inferences.
typescripttype ReadOnly<T> = { readonly [P in keyof T]: T[P] }; // Type `ReadOnlyUser` is inferred from `User` with all properties as readonly type User = { name: string; age: number }; type ReadOnlyUser = ReadOnly<User>;
typescripttype ReadOnly<T> = { readonly [P in keyof T]: T[P] }; // Type `ReadOnlyUser` is inferred from `User` with all properties as readonly type User = { name: string; age: number }; type ReadOnlyUser = ReadOnly<User>;
Type inference is a valuable tool in the TypeScript arsenal that helps developers write code that is both strongly typed and expressive. With advanced features like generics and mapped types, TypeScript's inference capabilities allow for a writing style that is concise yet powerful, forgoing the need for excessive annotations while still enforcing type safety. These advanced features illustrate the balance TypeScript strikes between the flexibility of JavaScript and the robustness of a statically typed language, making it an indispensable tool for modern web development.

Best practices for type inference in TypeScript projects
While type inference in TypeScript provides many benefits, developers must use it judiciously to maintain a codebase that is both easy to understand and to work with. Knowing when to rely on inference and when to annotate types explicitly can dramatically affect the readability and maintainability of the code. This section offers guidance on leveraging type inference to its full potential while avoiding potential pitfalls.
When to rely on inference
TypeScript's inference is most beneficial when it helps reduce the amount of boilerplate code without obscuring the intentions of the code. In scenarios such as local variables within a function where the type is clear from the value it's assigned, inference can safely be relied upon.
typescriptfunction calculateArea(radius: number) { const pi = 3.14159; // No need for `const pi: number = 3.14159;` return pi * radius * radius; }
typescriptfunction calculateArea(radius: number) { const pi = 3.14159; // No need for `const pi: number = 3.14159;` return pi * radius * radius; }
It is also appropriate to rely on type inference in complex generics where TypeScript can accurately deduce types based on usage. This can make generic utilities easier to work with and less verbose.
By allowing the compiler to infer types based on context, developers can focus on writing code without having to constantly update type declarations. This leads to faster development cycles, easier refactoring, and fewer opportunities for type-related errors, ultimately improving the overall maintainability of the codebase.
When to specify types explicitly
Explicit typing becomes important in scenarios where inferred types could be too broad or where future changes to the code could lead to unintentional errors. A common best practice is to annotate function return types in complex functions with multiple return types, which serves as documentation and ensures that changes within the function do not inadvertently alter the return type.
For public API surfaces of libraries or modules, explicitly stating types provides clear contracts to consumers of the API and can prevent inadvertent breaking changes.
typescript// Explicit return type for API surface function getItems(): Array<Item | string> { // implementation... }
typescript// Explicit return type for API surface function getItems(): Array<Item | string> { // implementation... }
Specifying types can also be important in class and interface definitions, where they ensure that the contracts defined by these constructs are clear and maintained throughout the lifetime of the application.
Balancing inference and explicit typing
Balancing inference and explicit typing in TypeScript is crucial for creating a codebase that is both concise and maintainable. While type inference can save time and make code more readable by allowing the compiler to deduce types automatically, there are instances where explicit typing is necessary to clarify intent and avoid ambiguity.
By using a balanced approach that combines inferred and explicit types, developers can leverage the strengths of TypeScript without cluttering the code with unnecessary type annotations. When dealing with complex type inferences, adding comments or using type aliases can provide additional documentation for future developers, making the reasoning behind certain type decisions more transparent.

Conclusion
TypeScript's type inference is a powerful tool that can reduce the boilerplate code typically associated with types in other languages. Understanding when and how to use type inference can lead to more concise and readable code. By following the best practices outlined in this post, developers can take full advantage of TypeScript's type inference capabilities to create robust, scalable applications, while keeping the codebase clean and maintainable.