Creating Accessible Form Components with React
Updated on · 9 min read|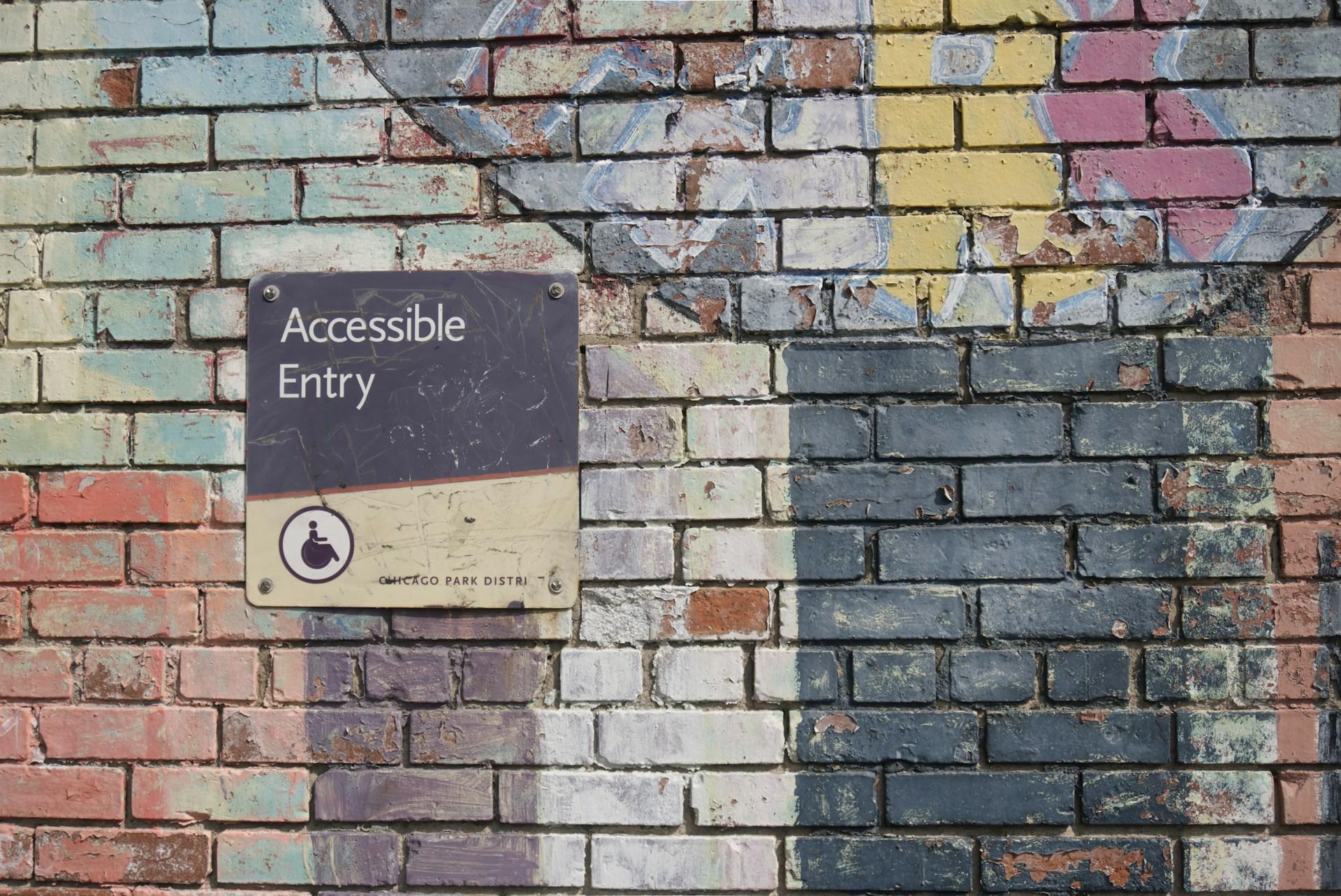
Creating web applications that are accessible to everyone, including individuals with disabilities, is not only a matter of legal compliance but also ethical practice. Accessible design ensures that all users have an equal opportunity to use and interact with web content.
In web development, forms are a critical component, serving as the main interface for user interaction and data input. However, forms can often become a barrier to users with disabilities if not designed thoughtfully. Given React's widespread usage and its component-based structure, developers should prioritize the creation of accessible forms.
This blog post aims to provide you with practical guidance on how to create form components that are inclusive and comply with accessibility standards using React. The post covers the core principles of accessibility, best practices for building accessible form components, and the tools and methods that can be used to enhance the accessibility of React form components.
Understanding accessibility in forms
Before going into the specifics of implementing accessible forms in React, it's important to clarify the core principles that guide web accessibility. The Web Content Accessibility Guidelines (WCAG) are the industry standard for building accessible web content.
The WCAG outlines a set of recommendations for making web content more accessible to a wider range of people with disabilities, including visual, auditory, physical, speech, cognitive, language, learning, and neurological disabilities. Following these guidelines not only benefits users with disabilities but also provides a better user experience for all.
Key principles of accessibility (POUR)
The WCAG is built around four fundamental principles, summarized by the acronym POUR, which stands for Perceivable, Operable, Understandable, and Robust:
- Perceivable - Users must be able to perceive the information being presented; it cannot be invisible to all of their senses.
- Operable - Users must be able to operate the interface; the interface cannot require interaction that a user cannot perform.
- Understandable - Users must be able to understand the information as well as the operation of the user interface.
- Robust - Users must be able to access the content as technologies advance. As web technologies evolve, the content should remain accessible.
Accessibility considerations for forms
Forms pose unique challenges for accessibility due to their need for user interaction. When considering accessibility for forms, developers need to keep a few key considerations in mind:
- Vision impairment - How will users who are blind or have low vision know what each field requires? Can they navigate through the form efficiently?
- Motor disabilities - Can users with limited dexterity navigate the form without a mouse, using only a keyboard or alternative input devices?
- Cognitive disabilities - Are the form instructions clear and simple to understand? Is the form layout consistent and predictable?
- Assistive technologies - How will screen readers and other assistive devices interpret the form elements and guide users through the form completion process?
By keeping these considerations in mind and adhering to the WCAG principles, you can design forms that accommodate users with a spectrum of abilities and preferences. In the next section, we'll explore how to apply these principles specifically within the context of React components.

Accessible form components in React
React's component-based architecture provides a powerful foundation for building accessible form components. By leveraging the right tools and following best practices, developers can create forms that are inclusive and compliant with accessibility standards, whether they are controlled or uncontrolled components.
Semantic HTML structure for form components
In React, using semantic HTML elements is fundamental for creating accessible form components. Semantic HTML not only improves the accessibility of forms but also enhances their overall structure, making them more maintainable and SEO-friendly.
When structuring forms, the following semantic elements can be used to improve accessibility and user experience:
form
- form components can be wrapped within aform
element to signify their collective purpose. This element defines the beginning and end of the form and ensures that browsers and assistive technologies recognize it as a form.
jsx<form onSubmit={handleSubmit}> {/* Form fields */} <button type="submit">Submit</button> </form>
jsx<form onSubmit={handleSubmit}> {/* Form fields */} <button type="submit">Submit</button> </form>
input
- theinput
element is used for various types of form controls, such as text inputs, checkboxes, radio buttons, and more. Thetype
attribute is used to define the input's purpose. Similarly,textarea
andselect
elements can be used for multi-line text inputs and dropdowns, respectively.
jsx<input type="text" id="username" name="username" />
jsx<input type="text" id="username" name="username" />
label
- thelabel
element is used to associate a text label with a form control. This improves the form's usability and accessibility by providing a clear description of the input field. Associating labels with inputs in React can be done in two ways: using thehtmlFor
attribute on the label which matches theid
attribute on the input, or by wrapping the input inside the label.
jsx<label htmlFor="username">Username:</label> <input type="text" id="username" name="username" />
jsx<label htmlFor="username">Username:</label> <input type="text" id="username" name="username" />
jsx<label> Username: <input type="text" name="username" /> </label>
jsx<label> Username: <input type="text" name="username" /> </label>
fieldset
andlegend
- thefieldset
element groups related form controls together, while thelegend
element provides a caption for thefieldset
. This is particularly useful for grouping related form fields and providing context for screen reader users, especially in large multistep or dynamic forms.
jsx<fieldset> <legend>Personal Information</legend> {/* Form fields */} </fieldset>
jsx<fieldset> <legend>Personal Information</legend> {/* Form fields */} </fieldset>
button
- thebutton
element is used to create a clickable button within a form. It can be used to submit the form, reset the form, or perform other actions. Thetype
attribute is used to specify the button's behavior, which issubmit
by default. In case the submit behavior is not desired, thetype
attribute should be set tobutton
to prevent accidental form submissions. That's why it's important to always specify thetype
attribute for the button element.
jsx<button>Submit</button>
jsx<button>Submit</button>
Here's a simple example that demonstrates the use of semantic HTML in a React functional component for a registration form, which is managed using React Hook Form:
jsximport React from "react"; import { useForm } from "react-hook-form"; function RegistrationForm() { const { register, handleSubmit } = useForm(); const onSubmit = (data) => { console.log(data); }; return ( <form onSubmit={handleSubmit(onSubmit)}> <fieldset> <legend>Personal Information</legend> <label htmlFor="firstName">First Name:</label> <input {...register("firstName")} type="text" id="firstName" /> <label htmlFor="lastName">Last Name:</label> <input {...register("lastName")} type="text" id="lastName" /> </fieldset> <fieldset> <legend>Account Details</legend> <label htmlFor="email">Email:</label> <input {...register("email")} type="email" id="email" /> <label htmlFor="password">Password:</label> <input {...register("password")} type="password" id="password" /> </fieldset> <button type="submit">Register</button> </form> ); }
jsximport React from "react"; import { useForm } from "react-hook-form"; function RegistrationForm() { const { register, handleSubmit } = useForm(); const onSubmit = (data) => { console.log(data); }; return ( <form onSubmit={handleSubmit(onSubmit)}> <fieldset> <legend>Personal Information</legend> <label htmlFor="firstName">First Name:</label> <input {...register("firstName")} type="text" id="firstName" /> <label htmlFor="lastName">Last Name:</label> <input {...register("lastName")} type="text" id="lastName" /> </fieldset> <fieldset> <legend>Account Details</legend> <label htmlFor="email">Email:</label> <input {...register("email")} type="email" id="email" /> <label htmlFor="password">Password:</label> <input {...register("password")} type="password" id="password" /> </fieldset> <button type="submit">Register</button> </form> ); }
In this example, the <fieldset>
and <legend>
elements group related fields together, while the <label>
and <input>
elements are used as per their semantic roles. By maintaining a semantic structure, the form is more intuitive for users and their assistive technologies, ensuring a more inclusive and accessible experience.
Importance of associating labels with input controls
In React, associating labels with their respective input controls through the htmlFor
and id
attributes ensures that forms are easy to navigate, both for users and assistive technologies. This is achieved by following the standard HTML practice of connecting a <label>
to an <input>
element, enhancing the interactive area, and making forms more accessible, especially for those with motor difficulties or touch device users:
jsx<label htmlFor="username">Username:</label> <input type="text" id="username" name="username"/>
jsx<label htmlFor="username">Username:</label> <input type="text" id="username" name="username"/>
One of the benefits of such an association is making the entire label clickable and increasing the target size. When the user clicks or taps on the label, the associated input field is focused, which aids in the navigation of the form and is especially useful for checkbox and radio button inputs with smaller clickable areas.
React Testing Library (RTL) complements this practice by providing tools that encourage accessibility. For instance, RTL allows querying the form elements by their accessible names, which are often derived from their associated labels. By incorporating RTL in our development workflow, we can write tests that mimic how a user interacts with the form, reinforcing the importance of properly associating labels with their controls.
To make the association between labels and inputs more convenient, it is often helpful to abstract this logic into a separate Field component, which will associate the label with the input control by default.
Understanding the role of placeholders
Placeholder text is used within form controls to provide hints or examples to users. However, they should not be used as replacements for labels, as this can lead to a variety of accessibility issues, such as poor color contrast when the placeholder text is light and the disappearance of instructions when users begin to type. Placeholders can also be missed by users of certain assistive technologies if not appropriately labeled. To use placeholders effectively in React without compromising accessibility, ensure they are combined with labels:
jsx<label htmlFor="message">Message:</label> <textarea id="message" name="message" placeholder="Type your message here…"></textarea>
jsx<label htmlFor="message">Message:</label> <textarea id="message" name="message" placeholder="Type your message here…"></textarea>

Keyboard navigation and focus management
Effective keyboard navigation and focus management are key to creating accessible web applications. Users with motor or visual impairments, who navigate primarily through the keyboard rather than a mouse, rely on thoughtful, consistent tab order and clear focus indicators. React's APIs, built on top of the native HTML functionality, provide a variety of ways to address accessibility concerns.
The tabIndex
attribute is integral to keyboard navigation. It determines the order in which elements receive focus as the user presses the Tab
key. A tabIndex
of 0
includes elements in the natural tabbing order, which is usually the order in which they appear in the document flow. This default behavior is recommended because it ensures a predictable navigation experience. A tabIndex
of -1
, while removing the element from the natural tab order, still allows it to receive programmatic focus, which can be useful for components that need to be focused in response to user actions but should not be tabbable during the regular navigation sequence.
However, developers should exercise caution with tabIndex
values greater than 0
. These can disrupt the document's natural tabbing order, leading to confusion and a frustrating experience for keyboard users. Instead of resorting to positive tabIndex
values, a better approach is normally to restructure the document or tweak the element presentation order via CSS while maintaining a logical tab order.
Immediate feedback on form validation and errors
Providing immediate and clear feedback on form validation and errors is a critical aspect of building accessible forms. Users need to know what went wrong and how to fix it, ideally without having to navigate away from the field where the error occurred. For users with assistive technologies, such as screen readers, using ARIA roles correctly is necessary to communicate these errors effectively.
When an error occurs, it should be announced to the user as soon as possible. This can be achieved using the ARIA role="alert"
attribute, which designates an element as an assertive live region. Screen readers will prioritize reading out any changes that occur within elements with an alert
role, ensuring that the message reaches the user promptly:
jsximport { useState } from "react"; function LoginForm() { const [error, setError] = useState(""); const handleSubmit = (event) => { event.preventDefault(); // Prevent form submission // Perform validation logic... // If an error is detected: setError("Invalid login credentials. Please try again."); // If no error, process form submission... }; return ( <form onSubmit={handleSubmit}> {/* Form fields go here */} {/* Display error message if an error exists */} {error && ( <div role="alert" aria-live="assertive" // Assistive technologies will announce this region's updates aria-atomic="true" // Announce the entire message, not just changed parts style={{ color: "red" }} // Red color for error text visibility > {error} </div> )} <button type="submit">Login</button> </form> ); }
jsximport { useState } from "react"; function LoginForm() { const [error, setError] = useState(""); const handleSubmit = (event) => { event.preventDefault(); // Prevent form submission // Perform validation logic... // If an error is detected: setError("Invalid login credentials. Please try again."); // If no error, process form submission... }; return ( <form onSubmit={handleSubmit}> {/* Form fields go here */} {/* Display error message if an error exists */} {error && ( <div role="alert" aria-live="assertive" // Assistive technologies will announce this region's updates aria-atomic="true" // Announce the entire message, not just changed parts style={{ color: "red" }} // Red color for error text visibility > {error} </div> )} <button type="submit">Login</button> </form> ); }
In this example, the setError
function is used to update the state with a relevant error message when the validation logic finds an issue with user input. The error state is then used to conditionally render a div with the alert role, which will trigger screen readers to announce the error message.

Conclusion
In conclusion, creating accessible forms with React is not just a matter of compliance, but an essential aspect of inclusive web development. By understanding the principles of accessibility and employing semantic HTML, proper ARIA roles, and React Testing Library alongside React Hook Form, we can build user-friendly, navigable, and compliant web applications.
This post covered important topics such as the proper association of labels with form controls, managing keyboard navigation and focus, providing clear feedback for validations, and the careful implementation of ARIA roles.
References and resources
- Build Dynamic Forms with React Hook Form
- Build a Multistep Form With React Hook Form
- Form Validation with React Hook Form
- Improving React Testing Library Tests
- Managing Forms with React Hook Form
- React Testing Library
- Simplifying Form Rendering In React with Field Component Abstraction
- Testing React Hook Form With React Testing Library
- Understanding Controlled vs Uncontrolled Components In React
- W3: Introduction to Understanding WCAG 2.0
- W3: Understanding the Four Principles of Accessibility